String Summary
Master string manipulation in Python with essential techniques like two-pointer, string reversal, and KMP algorithm. Perfect for coding interviews and real-world applications. Boost your skills today!
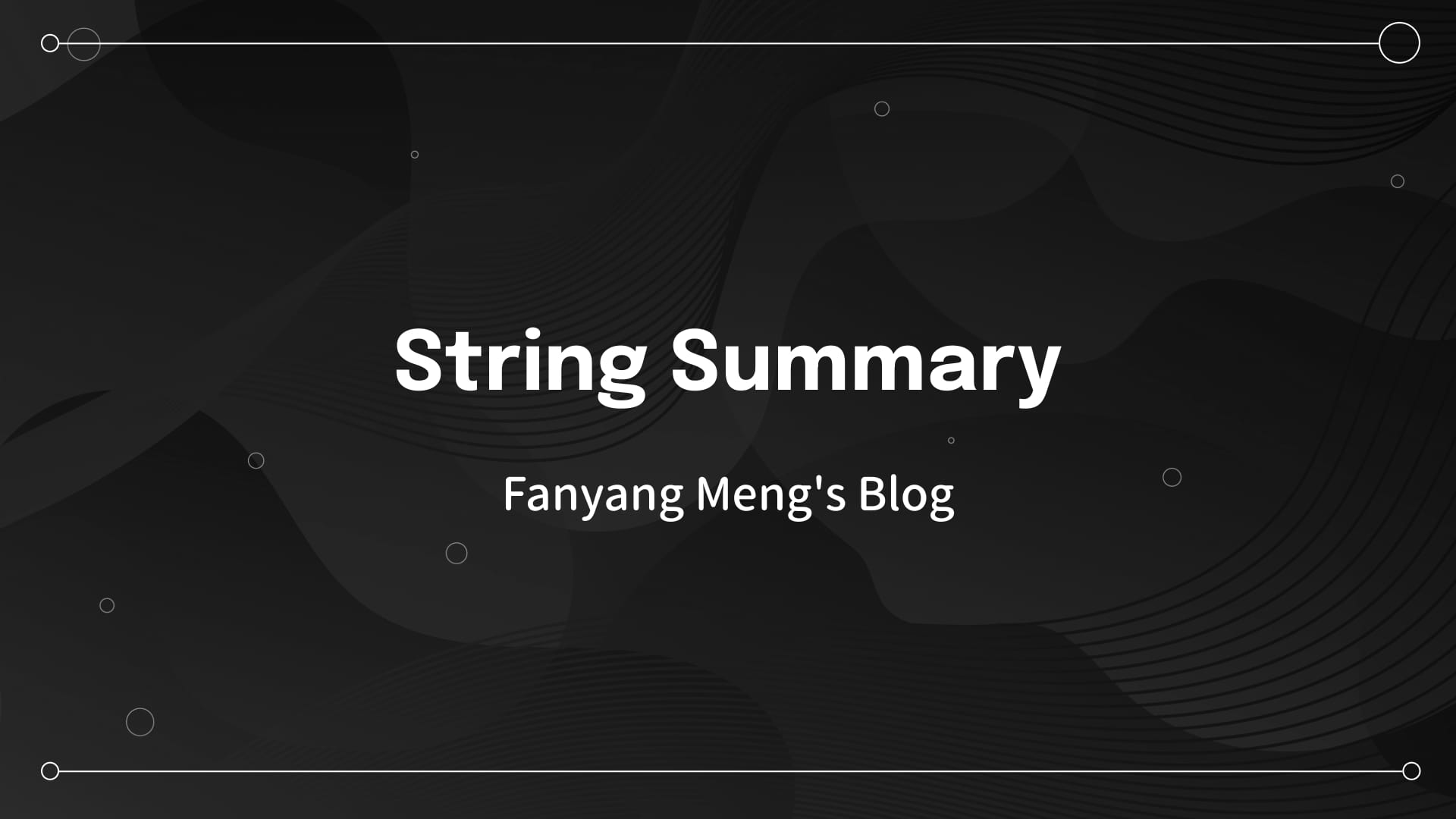
String manipulation is a fundamental skill in programming, encompassing everything from basic operations to complex pattern matching algorithms.
Understanding Strings in Python
In Python, strings are immutable sequences of characters. Unlike languages such as C that treat strings as character arrays, Python strings are objects with their own methods and properties. This makes string manipulation more intuitive and safer, though it comes with its own considerations for efficiency.
Key Features of Python Strings:
- Immutability: Strings cannot be modified after creation. Any operation that appears to modify a string actually creates a new one.
- Rich Built-in Methods: Python provides powerful methods like
split()
,join()
, and slicing for efficient string manipulation.
The Library Function Dilemma: When to Use Built-in Methods
When solving string-related problems, especially in coding interviews, a common question arises: Should you use built-in methods? Here’s a practical guideline:
Key Advice:
- Avoid built-in methods when they are central to the problem’s solution. For example:
- Don’t use
reversed()
when asked to implement string reversal. - Avoid
split()
when tasked with manually parsing strings.
- Don’t use
- Use built-in methods only when:
- They handle a minor part of the solution.
- You fully understand their implementation and time complexity.
- The problem doesn’t explicitly require implementing that functionality.
Essential String Manipulation Techniques
1. The Two-Pointer Technique
The two-pointer technique is a powerful approach for efficient string and array manipulation. It combines elegantly with Python’s features to solve complex problems with minimal space complexity.
Applications:
- Direct String/Array Operations: For problems like reversing a string (e.g., LeetCode 344. Reverse String), the two-pointer technique allows simultaneous operations from both ends, achieving O(n) time complexity and O(1) space complexity.
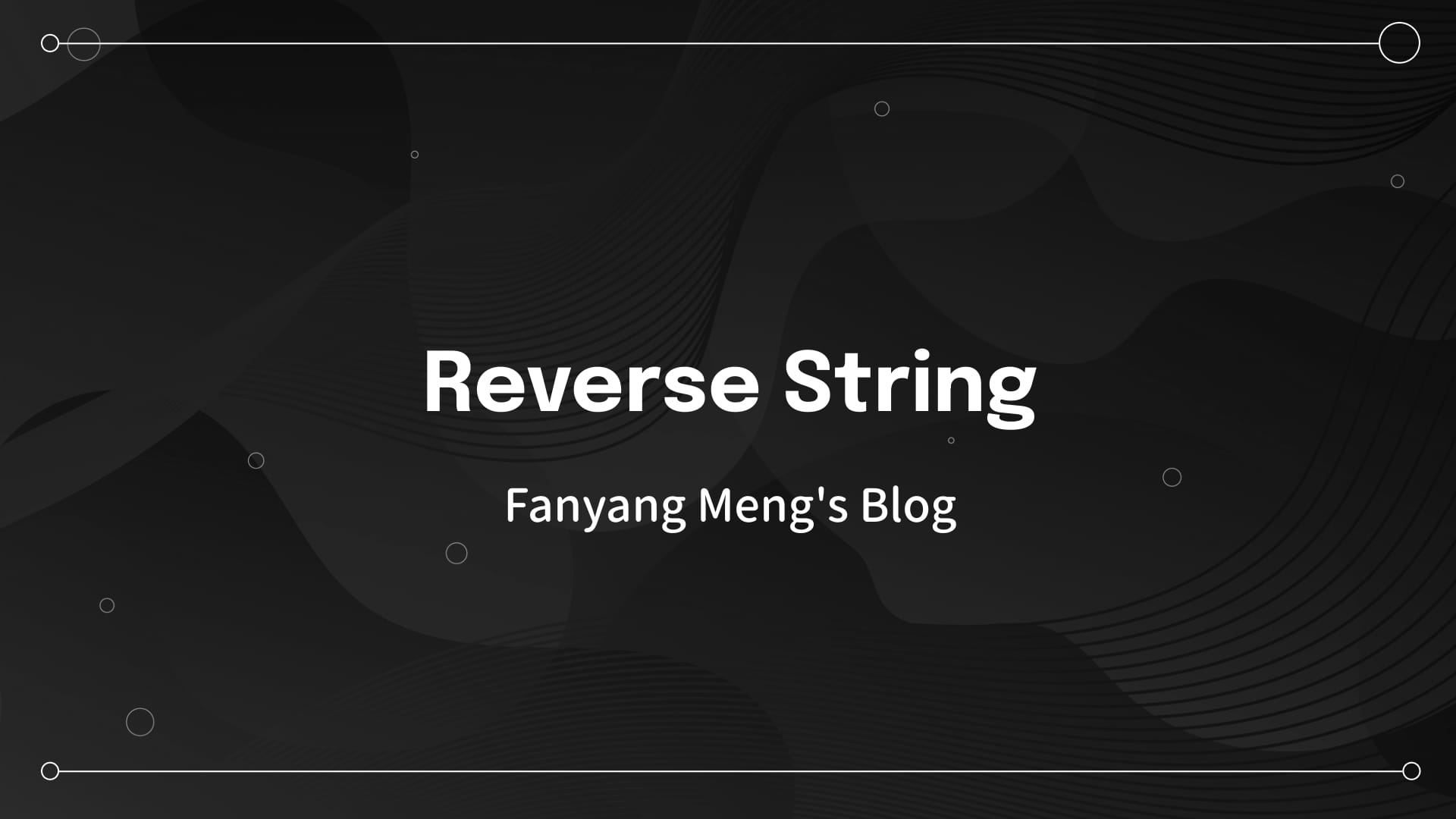
- Space-Optimized Operations: In array-filling problems, pre-calculate the final size, allocate space once, and use two pointers to fill from back to front, avoiding overwrites.
- Efficient Element Removal: For tasks like removing elements (e.g., LeetCode 27. Remove Element), this method prevents costly array shifts while maintaining array integrity.
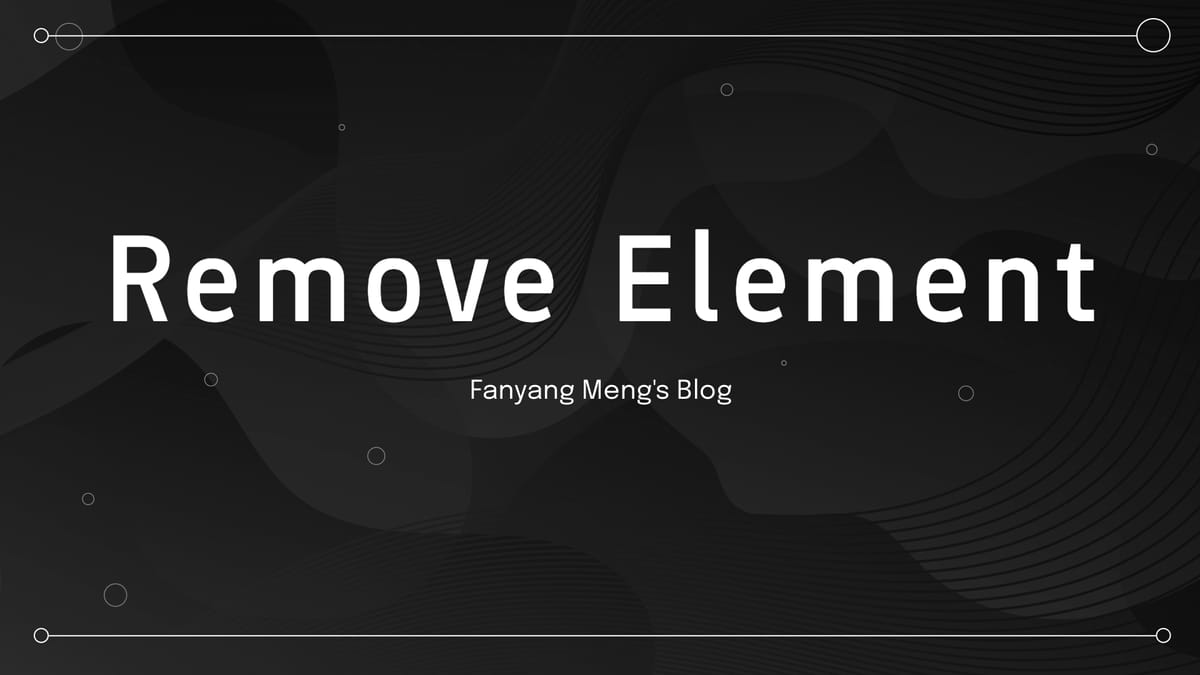
The same principle applies to problems like LeetCode 151. Reverse Words in a String, where redundant spaces are efficiently removed with O(n) time complexity.
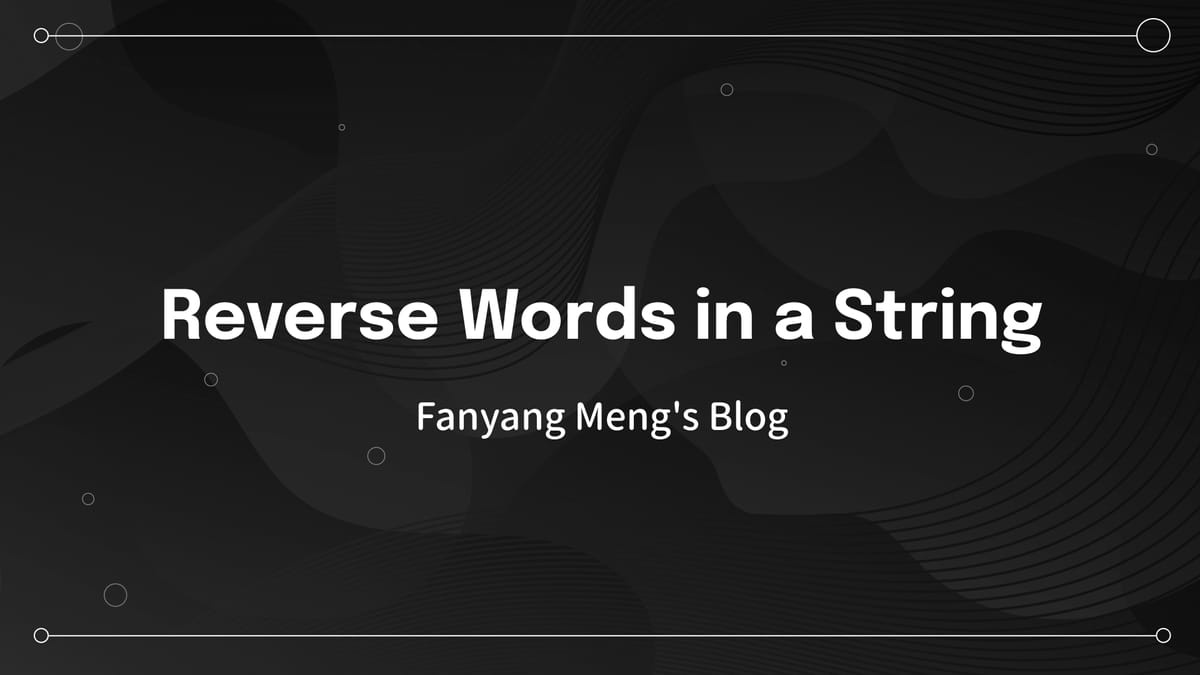
2. String Reversal
String reversal problems test your ability to simplify and optimize code while demonstrating strong programming logic. Here are some key strategies:
- Fixed Intervals or Patterns: In problems like LeetCode 541. Reverse String II, optimize loop logic by leveraging the increment step to process segments efficiently. For example:
- Use
i += 2 * k
to jump between segments. - Apply slicing (
s[i:i + k]
) to handle only the relevant part for reversal.
- Use
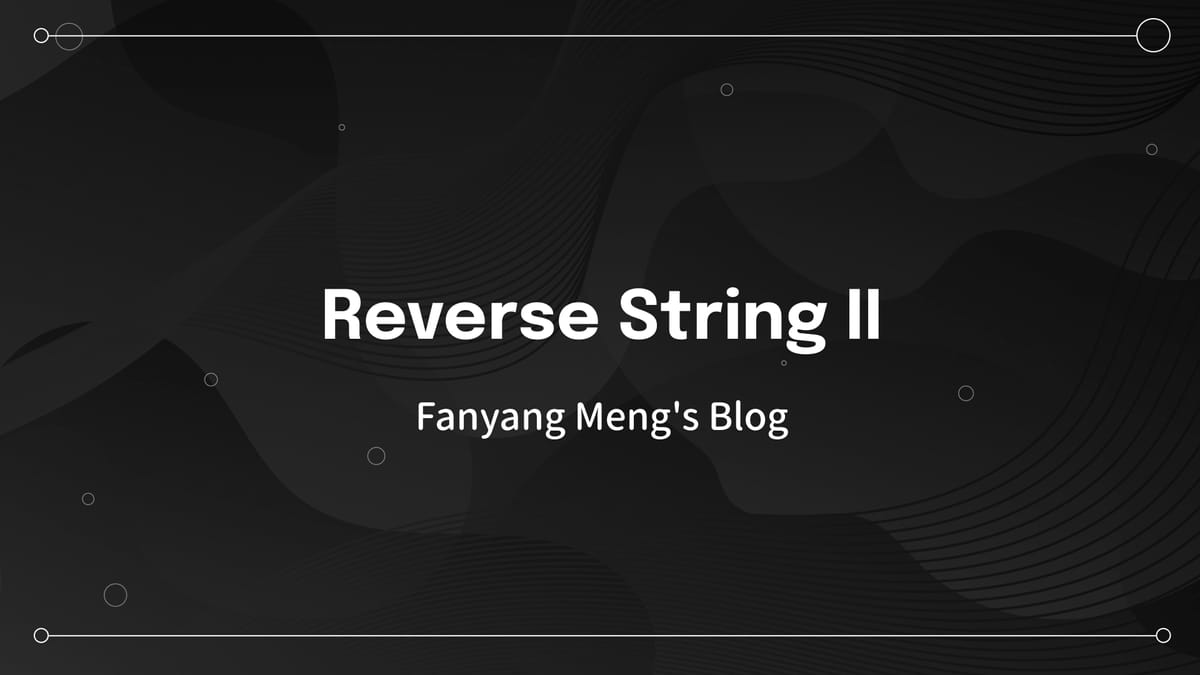
- Reverse Words in a String: Achieve word reversal by first reversing the entire string and then reversing each word individually.
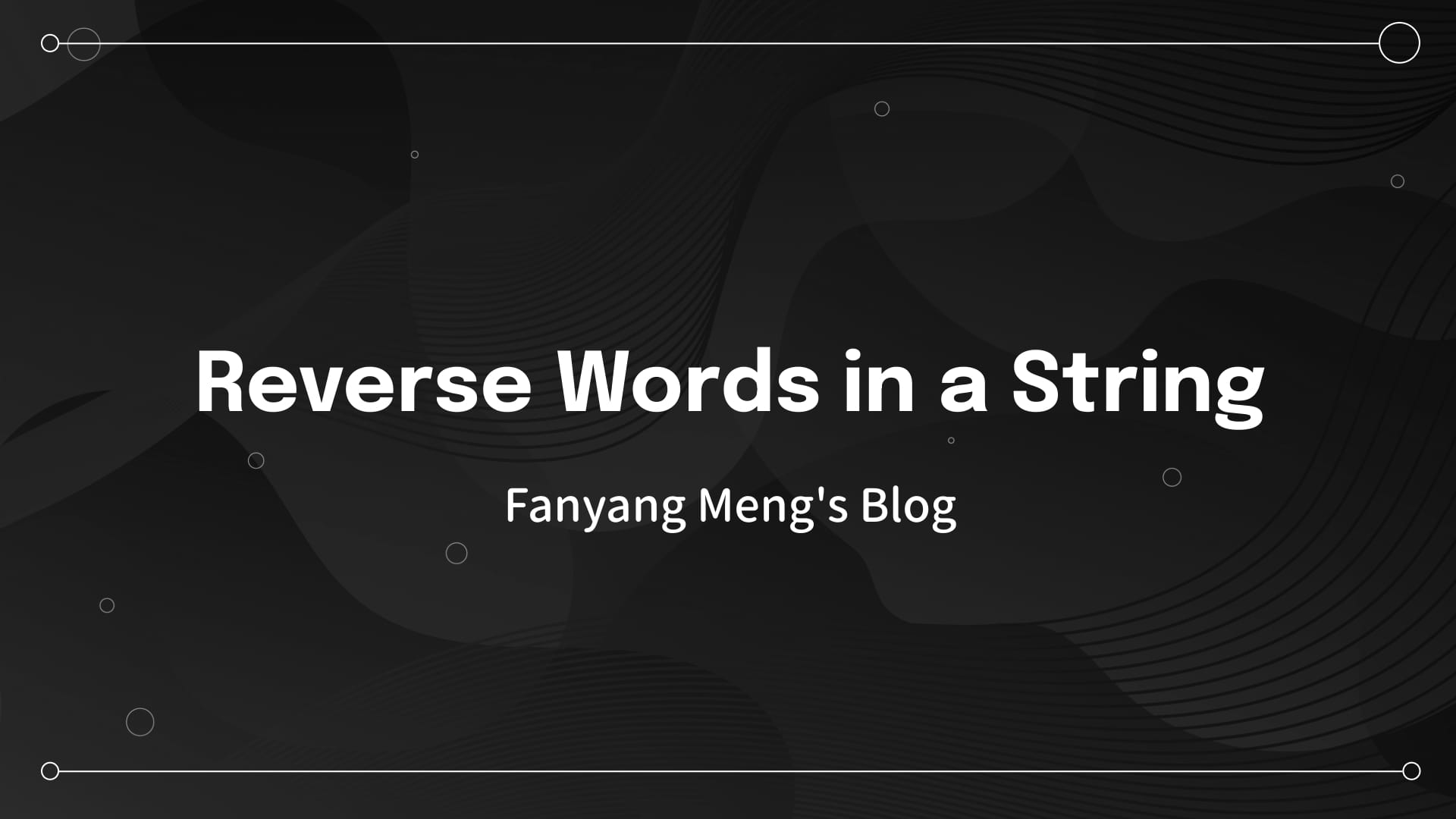
3. The Knuth-Morris-Pratt (KMP) Algorithm
The KMP algorithm is a highly efficient pattern-matching tool. Its strength lies in leveraging information from previous mismatches to skip redundant comparisons, significantly speeding up the matching process.
Core Concepts:
- Prefix Table: The heart of KMP, this table identifies how much of the pattern can be reused after a mismatch. It represents the length of the longest prefix that is also a suffix for substrings of the pattern.
- Prefix: A substring starting from the first character (excluding the last).
- Suffix: A substring ending at the last character (excluding the first).
For example, the pattern ababc
has a prefix table [0, 0, 1, 2, 0]
. At index i
, prefix[i]
indicates how many characters can be skipped due to previously matched prefixes.
Why Use the Prefix Table?
- Efficient Backtracking: Instead of restarting from the beginning, KMP uses the prefix table to determine where to resume matching, avoiding unnecessary recomparisons.
Python Implementation of the Prefix Table
def build_prefix_table(pattern: str) -> list[int]:
"""
Builds the prefix table for KMP algorithm.
Args:
pattern: The string pattern to search for
Returns:
A list of integers representing the longest proper prefix that is also a suffix
"""
pattern_length = len(pattern)
prefix_table = [0] * pattern_length
prev_prefix_length = 0
current_pos = 1
while current_pos < pattern_length:
if pattern[current_pos] == pattern[prev_prefix_length]:
prev_prefix_length += 1
prefix_table[current_pos] = prev_prefix_length
current_pos += 1
else:
if prev_prefix_length != 0:
prev_prefix_length = prefix_table[prev_prefix_length - 1]
else:
prefix_table[current_pos] = 0
current_pos += 1
return prefix_table
Applications of KMP
KMP can solve two classic problems efficiently:
- Substring Search: 28. Find the Index of the First Occurrence in a String
Use KMP to determine if a substring exists in a string and find its starting position.
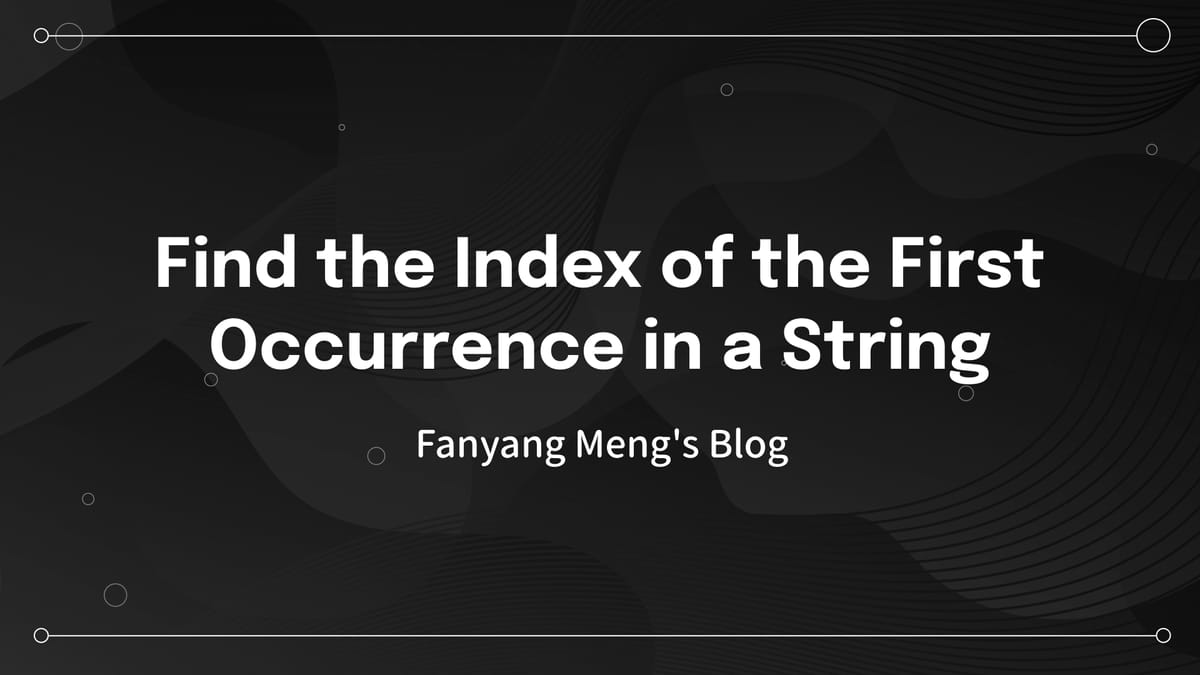
- Repeated Substring Pattern: 459. Repeated Substring Pattern
Use KMP to check if a string is constructed by repeating a substring.
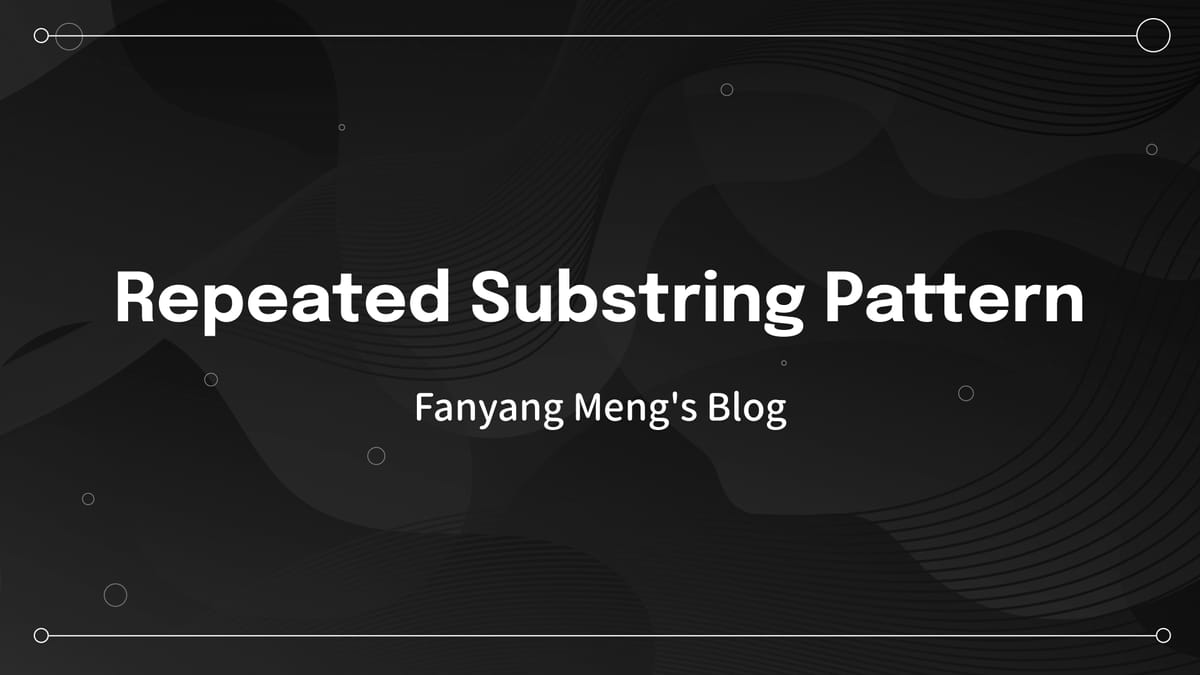
Conclusion
Mastering string manipulation in Python requires a solid understanding of both fundamental concepts and advanced techniques. By leveraging tools like the two-pointer technique, efficient string reversal strategies, and the KMP algorithm, you can tackle a wide range of problems with confidence. Whether you're preparing for coding interviews or working on real-world applications, these skills will prove invaluable.
Discussion