Stacks and Queues Summary
This blog post provides a summary of stacks and queues in Python, covering their implementations, applications, and optimization strategies. Explore efficient solutions for classic problems about stacks and queues with Python examples and expert tips.
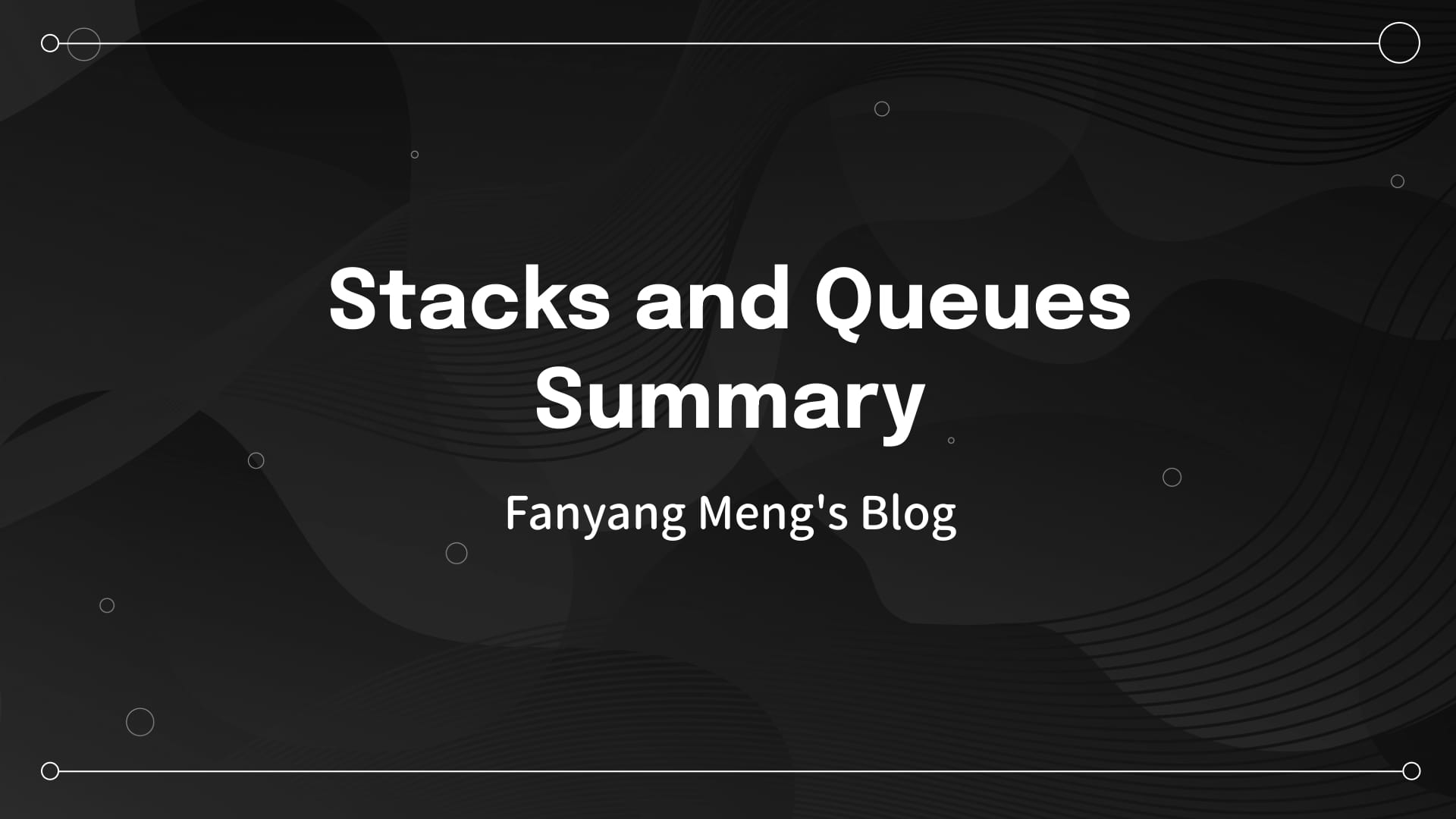
Fundamentals of Stacks and Queues
Stacks and queues are foundational data structures essential for solving numerous computational problems. A stack operates on the Last In, First Out (LIFO) principle, while a queue follows the First In, First Out (FIFO) principle.
To better understand their implementation and applications, I’ve outlined a few critical questions to consider, specifically in Python:
- Is the
list
in Python inherently a stack structure? - Does Python's built-in
list
or other modules use any specific underlying implementation for stacks and queues? - How are stacks and queues implemented in Python's standard library?
- Can we iterate over stacks and queues in Python?
Implementing Stacks and Queues in Python
Python provides various ways to implement stacks and queues:
Stacks
Using collections.deque
: For optimized stack operations, collections.deque
is preferred as it offers efficient appends and pops from both ends.
from collections impor deque
stack = deque()
stack.append(1) # Push 1
stack.append(2) # Push 2
stack.pop() # Pop 2 (LIFO)
Using list
: Python’s built-in list
allows stack operations using the append()
method for pushing and pop()
for removing the last element.
stack = []
stack.append(1) # Push 1
stack.append(2) # Push 2
stack.pop() # Pop 2 (LIFO)
Queues
Using list
: A queue can be implemented with list
using append()
for enqueuing and pop(0)
for dequeuing. However, this approach is inefficient for large datasets.
queue = []
queue.append(1) # Enqueue 1
queue.append(2) # Enqueue 2
queue.pop(0) # Dequeue 1 (FIFO)
Using collections.deque
: The deque
data structure is highly efficient for queue operations.
from collections import deque
queue = deque()
queue.append(1) # Enqueue 1
queue.append(2) # Enqueue 2
queue.popleft() # Dequeue 1 (FIFO)
Using queue.Queue
: For thread-safe queues, the queue.Queue
module is ideal.
from queue import Queue
queue = Queue()
queue.put(1) # Enqueue 1
queue.put(2) # Enqueue 2
queue.get() # Dequeue 1 (FIFO)
Using multiprocessing.Queue
: To handle inter-process communication, use multiprocessing.Queue
.
from multiprocessing import Queue
queue = Queue()
queue.put(1) # Enqueue 1
queue.put(2) # Enqueue 2
queue.get() # Dequeue 1 (FIFO)
For more details, see Fundamentals of Stacks and Queues.
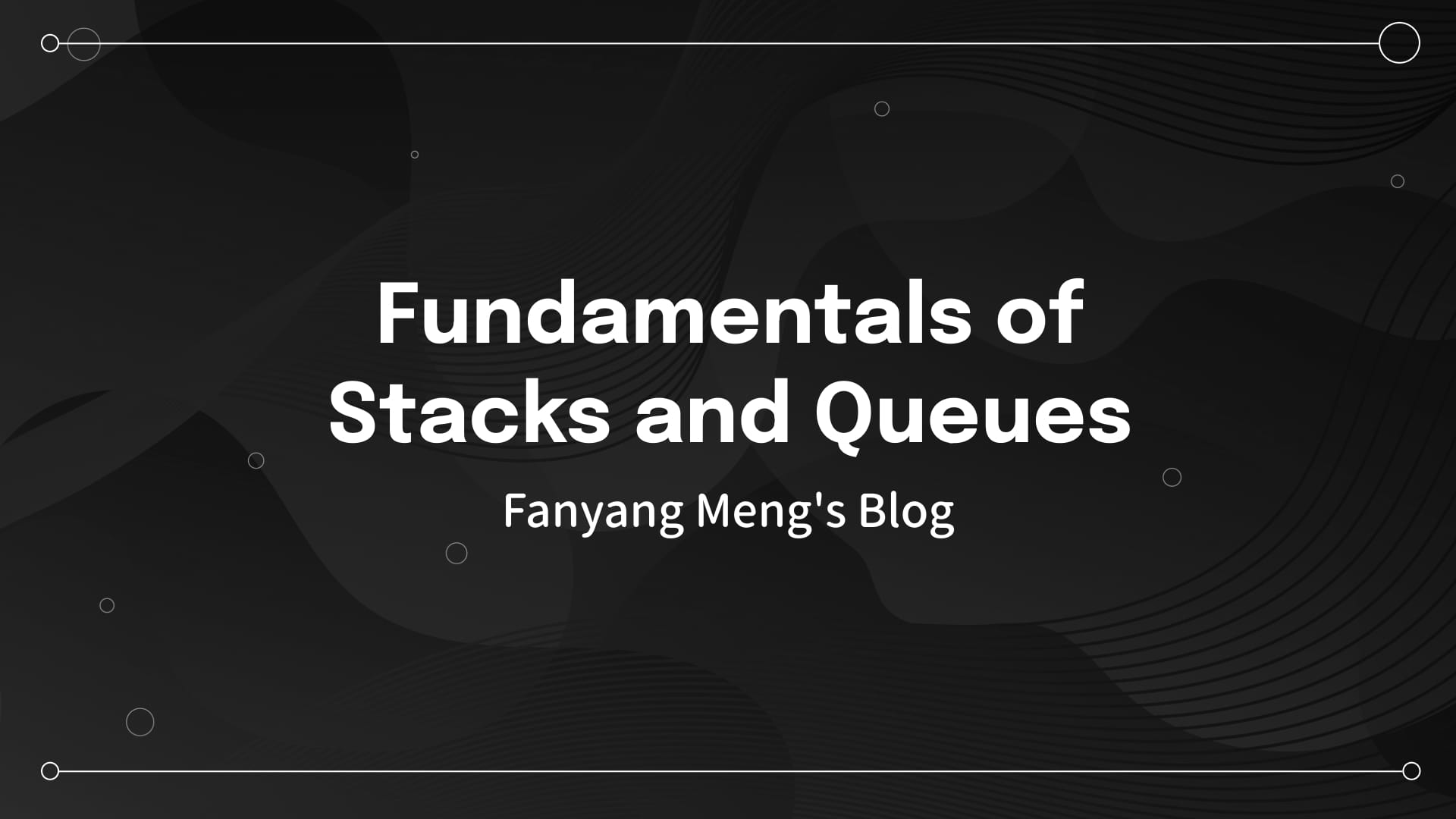
Classic Stack Problems
Bracket Matching Problem
In 20. Valid Parentheses , we explored how stacks perfectly solve bracket matching problems.
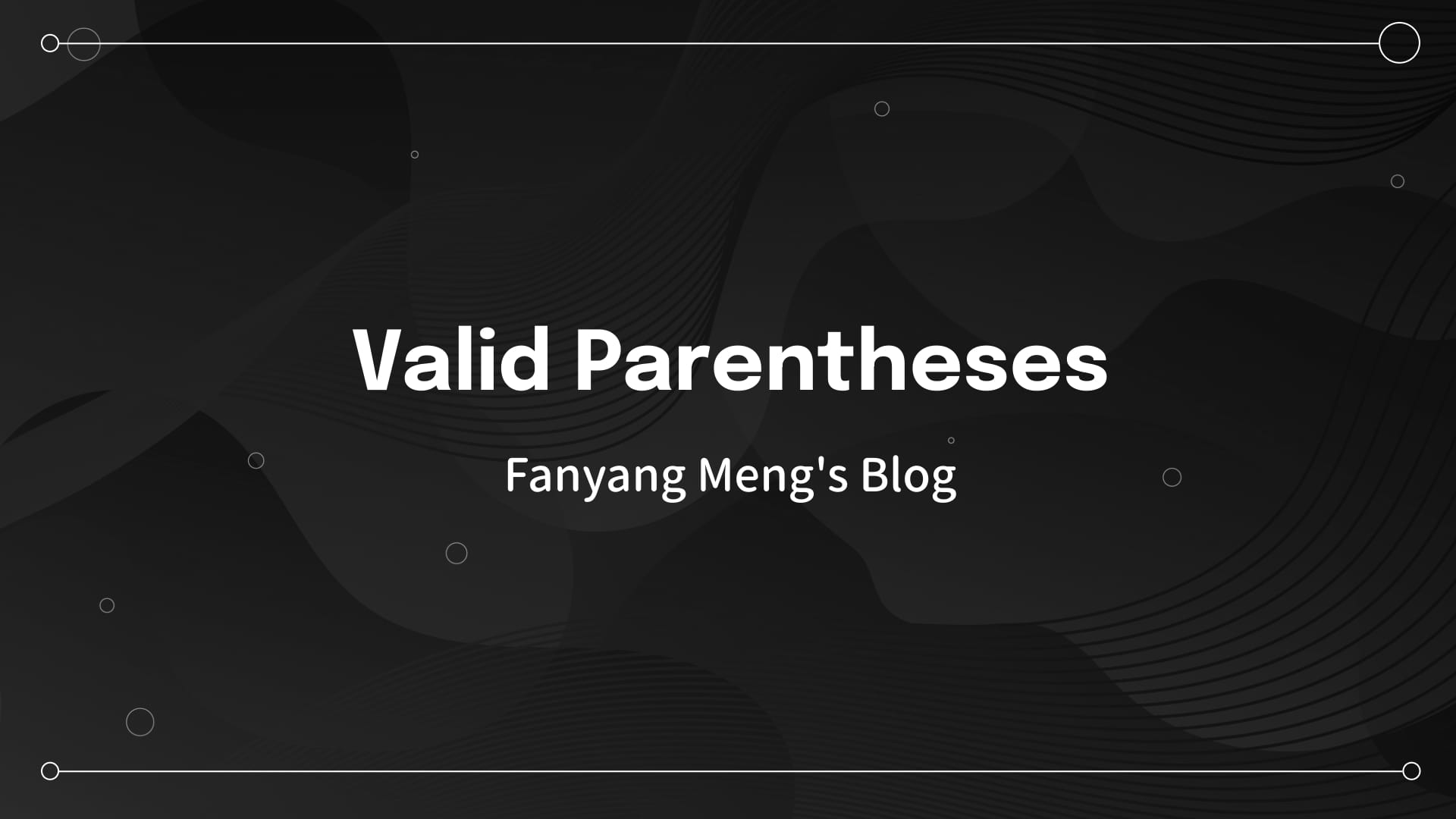
Three common mismatching scenarios:
- Extra left brackets
- Mismatched bracket types
- Extra right brackets
The idea is to push opening brackets onto the stack and pop them when a closing bracket is encountered. If the stack is empty or mismatched at any point, the sequence is invalid.
String Deduplication
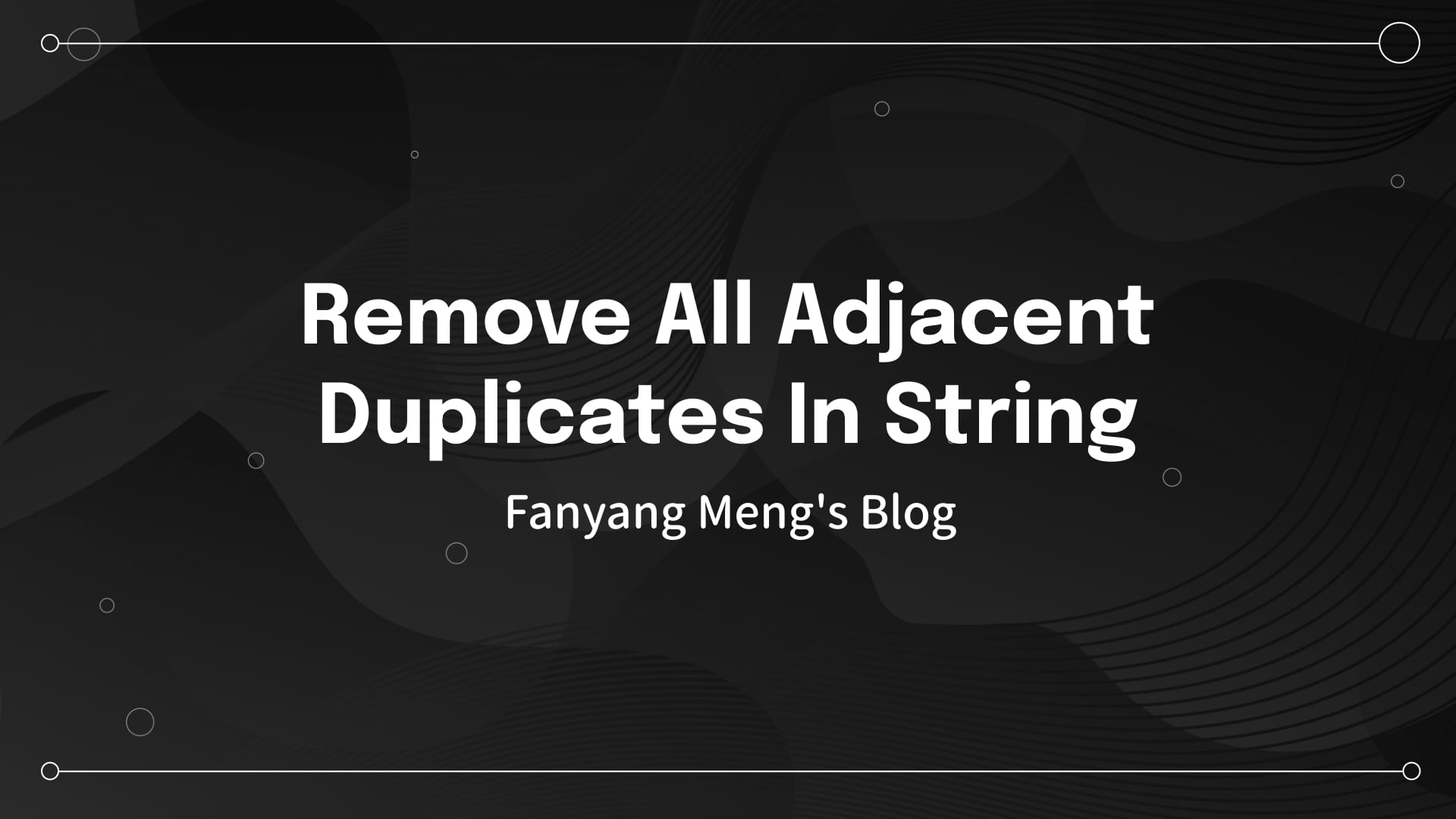
The 1047. Remove All Adjacent Duplicates In String problem shows how stacks can elegantly handle string processing. By pushing characters onto a stack and popping when duplicates are found, we can efficiently remove adjacent duplicates.
Reverse Polish Notation (RPN)
In 150. Evaluate Reverse Polish Notation , we see how stacks handle mathematical expressions. The solution pattern resembles the string deduplication problem, processing elements sequentially while maintaining necessary state.
Classic Queue Problems
Sliding Window Maximum
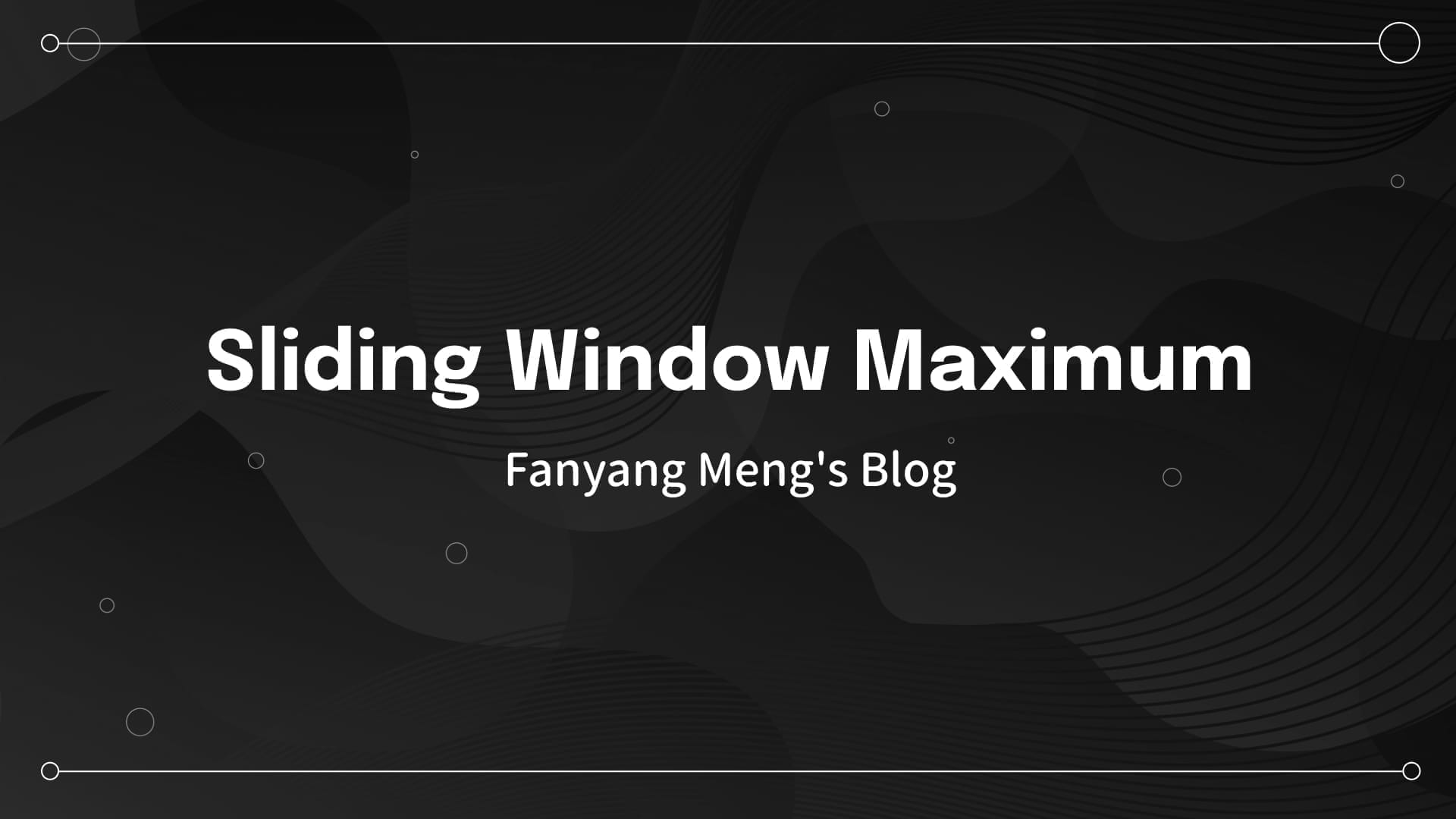
In 239. Sliding Window Maximum , we introduced an important data structure: the monotonic queue. This problem can be tricky at first and often requires multiple reviews to fully grasp the concept.
The key insight is that we don't need to keep all elements in the sliding window. We only need to maintain elements that could potentially become the maximum value in the window, while ensuring these elements are stored in descending order.
This queue that maintains elements in a monotonically decreasing (or increasing) order is called a monotonic queue. In Python, while we don't have a built-in monotonic queue, we can implement one using collections.deque
.
It's important to note that a monotonic queue is not simply sorting the elements in the window - that would be equivalent to using a priority queue, which is a different approach with different characteristics.
When designing a monotonic queue, the pop
and push
operations should follow these rules:
pop(value)
: If the value being removed from the window equals the element at the front of the monotonic queue, remove that element. Otherwise, no operation is needed.push(value)
: If the value being pushed is larger than elements at the back of the queue, keep removing elements from the back until either the queue is empty or we find an element larger than or equal to the value being pushed.
By following these rules, whenever the window slides, we can simply check the front of our monotonic queue to get the maximum value in the current window.
It's important to understand that this specific implementation of push and pop operations is tailored for the sliding window maximum problem.
The concept of a monotonic queue is flexible and can be implemented differently for different scenarios. The key principle is maintaining the monotonic (increasing or decreasing) property of the elements in the queue.
We use Python's collections.deque
as the underlying data structure because it supports efficient operations at both ends of the queue, which is perfect for implementing a monotonic queue. The deque
structure allows us to add or remove elements from either end in O(1) time.
Top K Frequent Elements
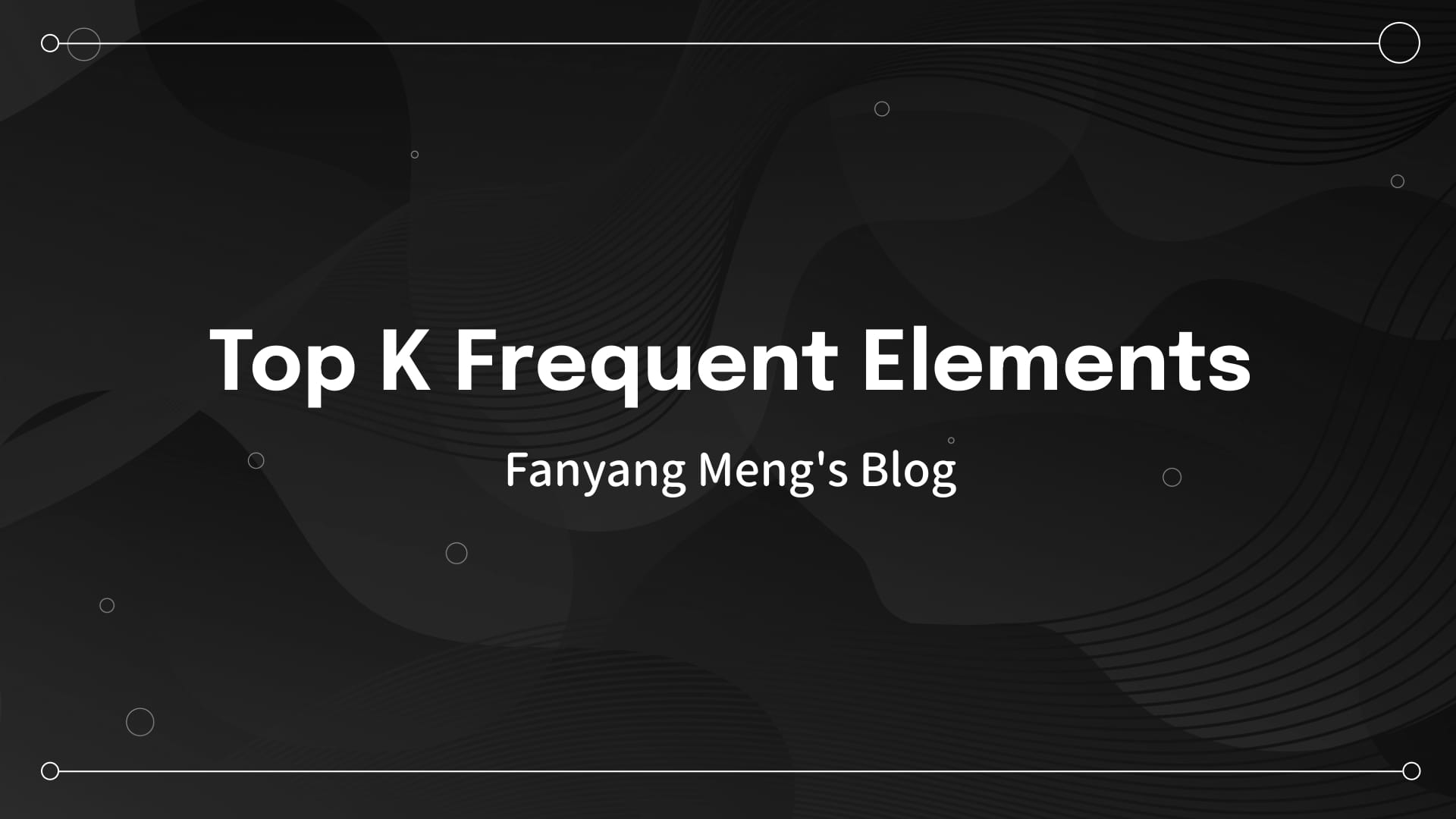
In 347. Top K Frequent Elements, we explore the relationship between finding top K elements and priority queues.
What is a Priority Queue?
A priority queue is essentially a heap disguised as a queue. While it looks like a queue from the outside with operations to add elements at one end and remove them from the other, its internal structure is quite different from a regular queue.
The key feature of a priority queue is that its elements are automatically ordered according to their priority (or value). In Python, we implement priority queues using the heapq
module, which provides an implementation of the heap queue algorithm.
Understanding Heaps
A heap is a complete binary tree with a special property: each node's value is either greater than or equal to (max-heap) or less than or equal to (min-heap) the values of its children.
There are two types of heaps:
- Max Heap: Parent nodes are greater than or equal to their children (root is the maximum element)
- Min Heap: Parent nodes are less than or equal to their children (root is the minimum element)
In Python, heapq
implements a min-heap by default. Here's how you can work with it:
import heapq
# Creating a min heap
min_heap = []
heapq.heappush(min_heap, 3)
heapq.heappush(min_heap, 1)
heapq.heappush(min_heap, 4)
print(heapq.heappop(min_heap)) # Outputs: 1 (smallest element)
# Creating a max heap (by negating values)
max_heap = []
heapq.heappush(max_heap, -3)
heapq.heappush(max_heap, -1)
heapq.heappush(max_heap, -4)
print(-heapq.heappop(max_heap)) # Outputs: 4 (largest element)
For the Top K elements problem, we use a priority queue to partially sort the frequency data. This is more efficient than sorting all elements because we only need the K most frequent ones.
The time complexity is O(n log k) rather than O(n log n) for a full sort, because we're only maintaining a heap of size k. This is a significant optimization when k is much smaller than n!
Remember: While Python's heapq
provides a min-heap implementation, you can easily adapt it for max-heap operations by negating values or using custom comparison functions. The module also provides convenient functions like heapq.nlargest()
and heapq.nsmallest()
for finding top K elements directly.
Conclusion
Throughout this exploration of stacks and queues in Python, we've covered essential implementations, classic problems, and specialized variations of these fundamental data structures. Let's recap the key insights:
Implementation Flexibility
Python offers multiple ways to implement stacks and queues, each with its own advantages:
collections.deque
for efficient general-purpose stacks and queues- Built-in
list
for simple stack operations queue.Queue
for thread-safe operationsmultiprocessing.Queue
for inter-process communication
Problem-Solving Applications
We've seen how these structures solve various algorithmic problems:
- Stacks excel at parsing problems (bracket matching) and tracking state (string deduplication)
- Queues, particularly specialized variants like monotonic queues, efficiently handle sliding window problems
- Priority queues (heaps) optimize partial sorting problems like finding top K elements
Remember that while Python provides high-level abstractions for these data structures, understanding their underlying mechanics and appropriate use cases is crucial for writing efficient and maintainable code. The key is not just knowing how to use these structures, but understanding when and why to use each variant.
Discussion