Hash Table Summary
Explore hash tables in Python: from fundamentals to practical applications. Learn when to use lists, sets, and dictionaries effectively with real coding examples. Master efficient problem-solving techniques for algorithms like Two Sum, Valid Anagram, and Happy Number.
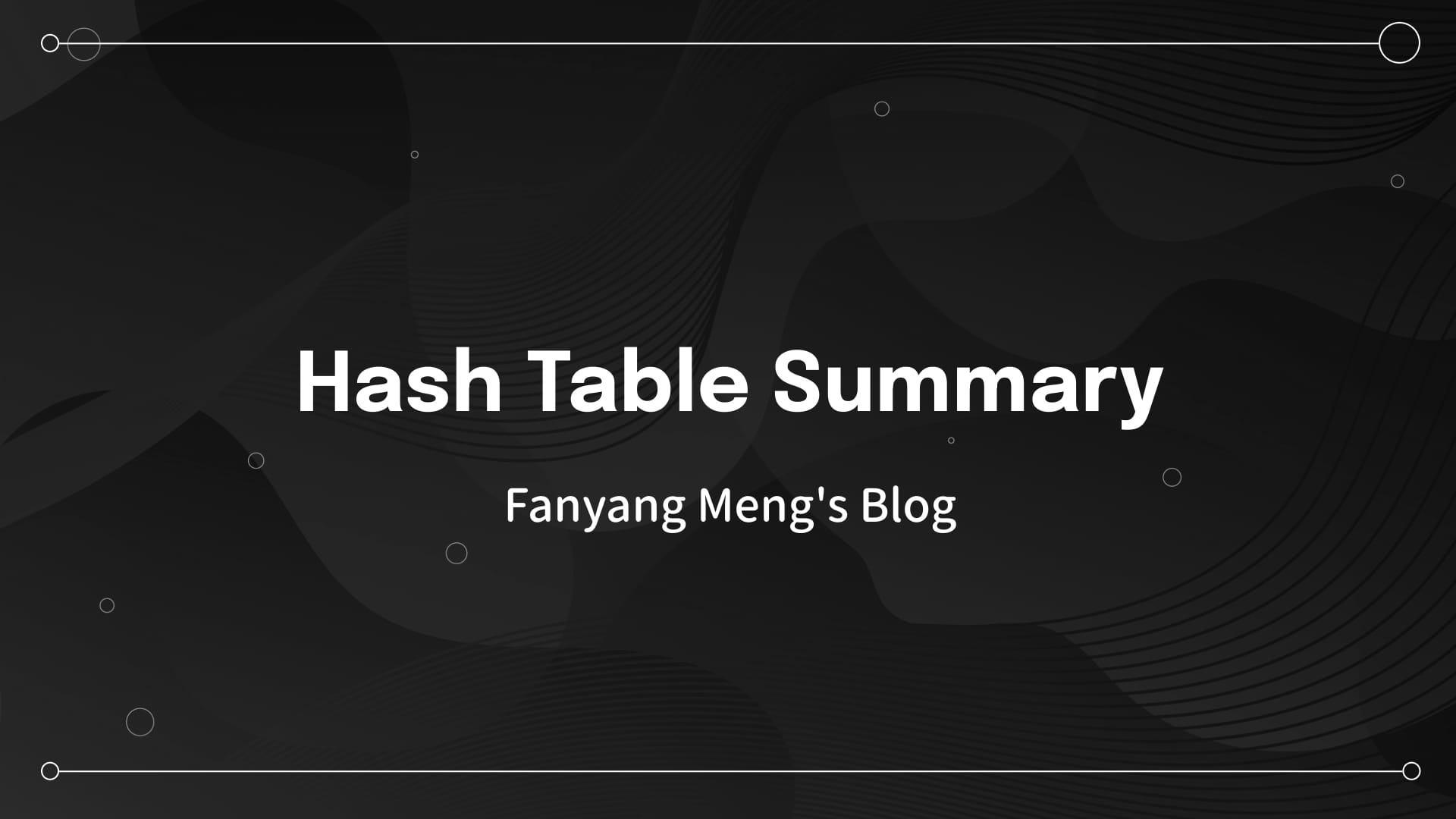
Hash tables are a fundamental data structure widely used to quickly determine if an element exists in a collection. In this post, we’ll provide a structured overview of hash tables, exploring their theoretical underpinnings, practical applications, and implementation nuances. By the end, you’ll have a solid understanding of when and how to use arrays, sets, and maps as hash table structures.
1. Fundamentals of Hash Tables
Hash tables rely on hash functions and mechanisms to handle hash collisions.
- Hash Function: Maps a key to an index in the hash table.
- Hash Collisions: Occur when multiple keys map to the same index. Python’s built-in hash-based structures like
set
anddict
manage collisions internally for you.
Common Hash Table Structures in Python
- Lists
- Sets
- Dictionaries
These structures are efficient and easy to use in Python:
- Lists can simulate hash tables for specific scenarios, but they require manual handling of keys (e.g., via indices).
- Sets provide a simple way to store unique elements and allow fast membership testing.
- Dictionaries (
dict
) map keys to values, offering flexible and efficient key-value pair management.
Understanding when to use each structure ensures efficient and effective problem-solving.
2. Classic Hash Table Applications
Using Lists as Hash Tables
Lists are ideal when the problem domain is small and constrained.
Example 1: Valid Anagram
- Problem: Check if two strings are anagrams.
- Solution: Use a list to count character frequencies, as the problem only involves lowercase letters.
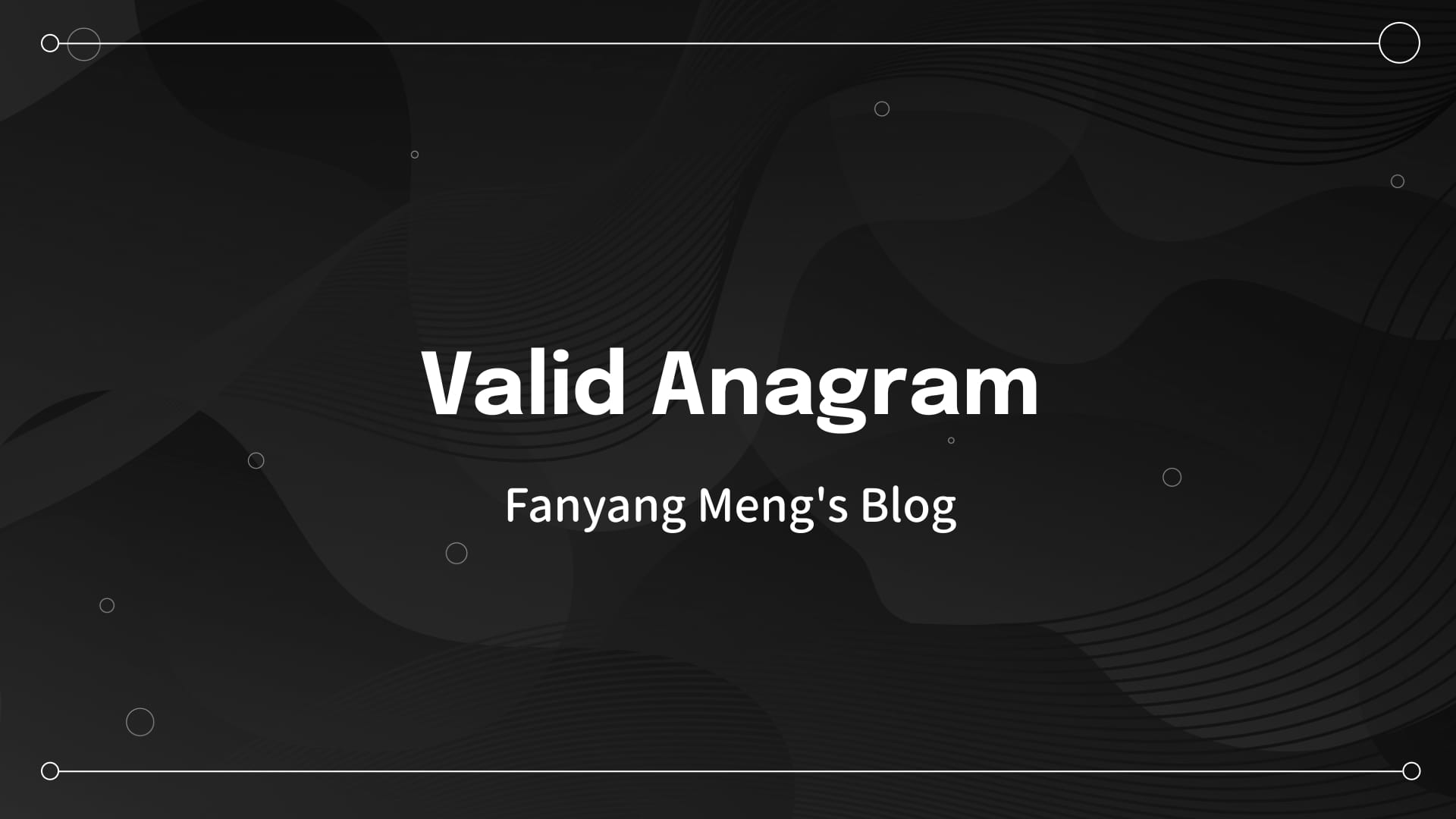
Example 2: Ransom Note
- Problem: Determine if one string can be constructed using another string.
- Solution: Similar to the anagram problem, a list efficiently tracks letter counts.
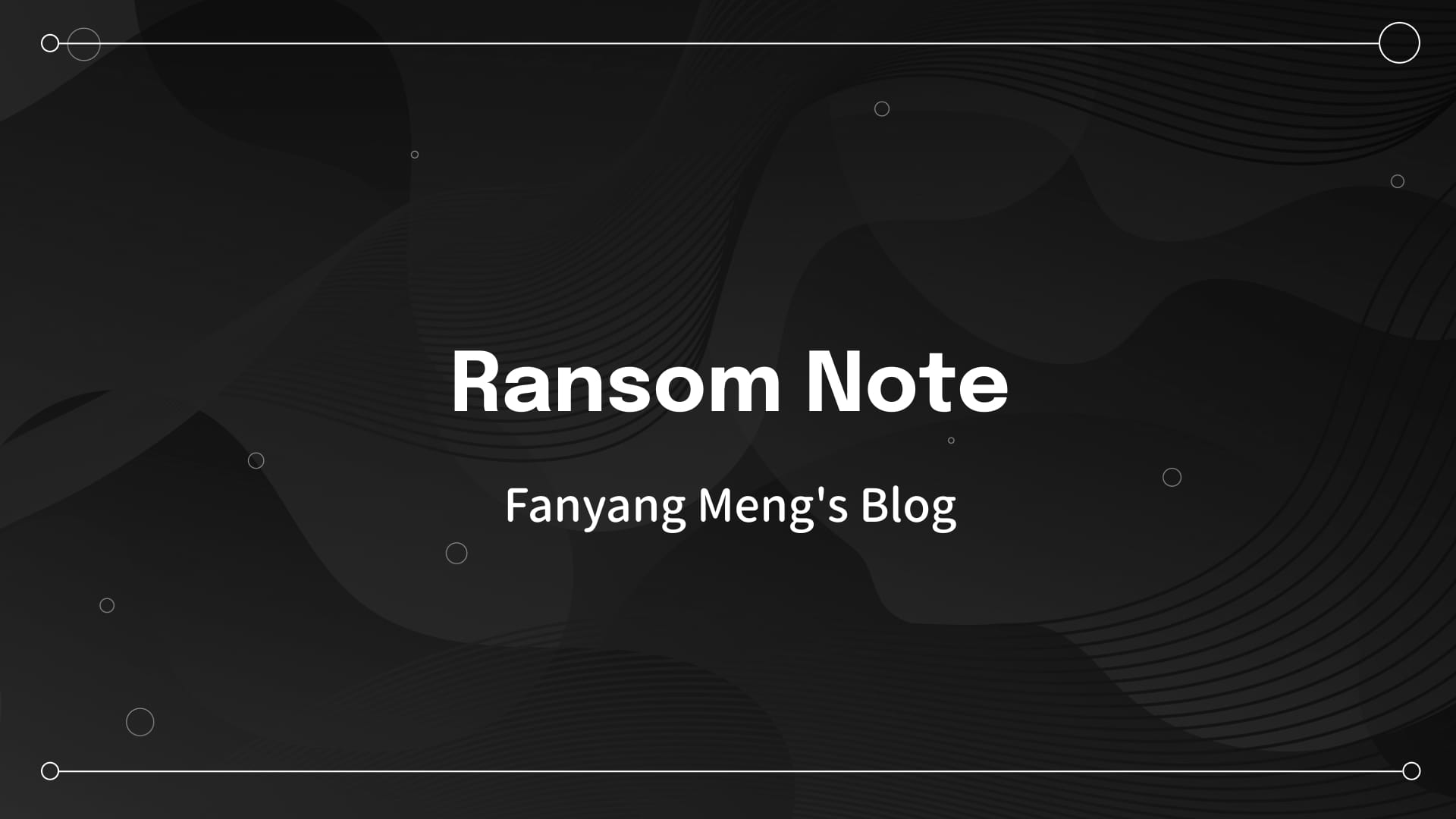
Why not use dictionaries here? While dictionaries can solve these problems, lists are more space-efficient when the domain is constrained (e.g., lowercase letters).
Using Sets as Hash Tables
Sets are ideal when you need to store unique values and perform fast membership checks.
Example 3: Intersection of Two Arrays
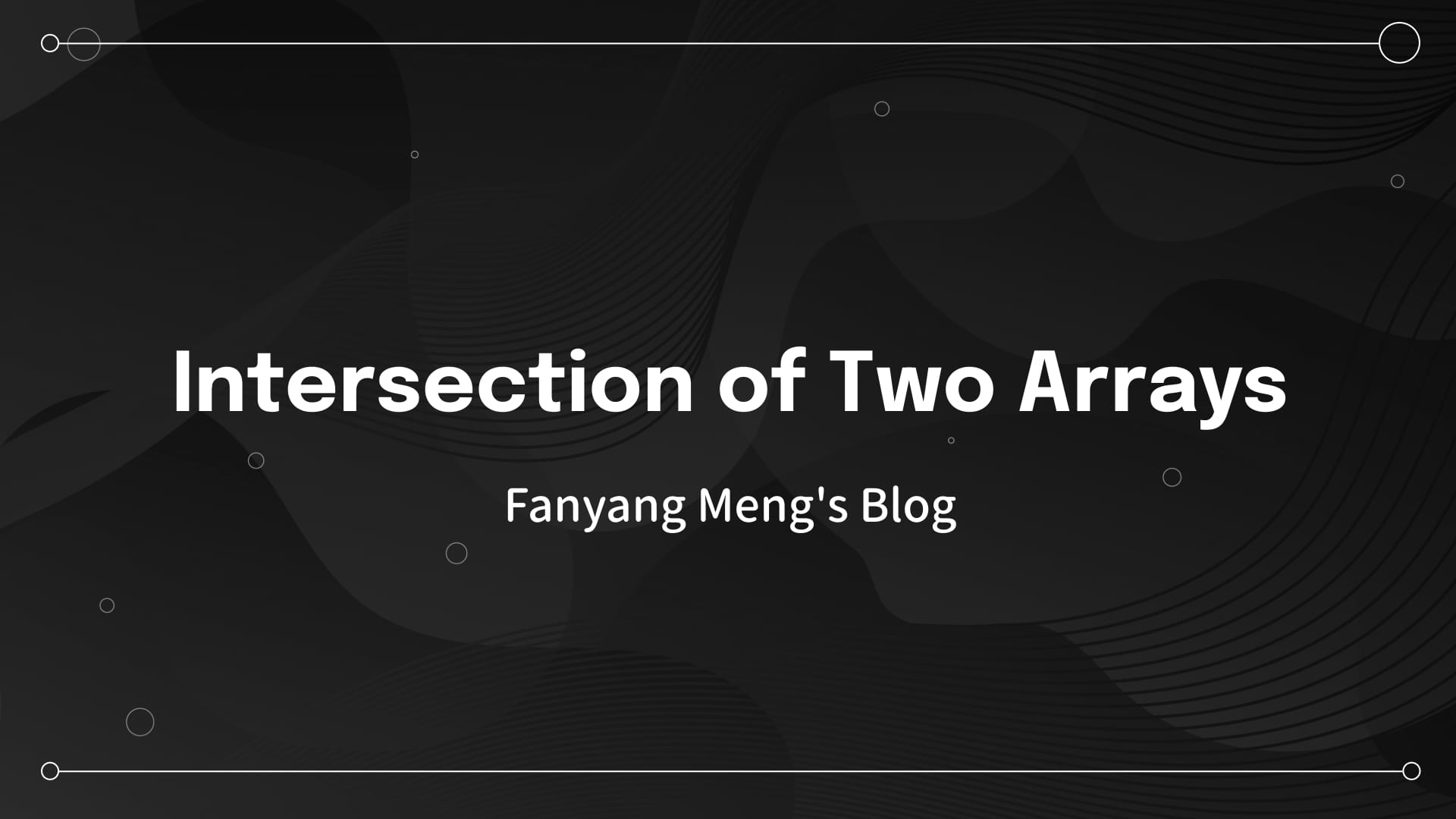
- Problem: Find the intersection of two arrays.
- Solution: Use a set to track unique values and check for intersections.
Example 4: Happy Number
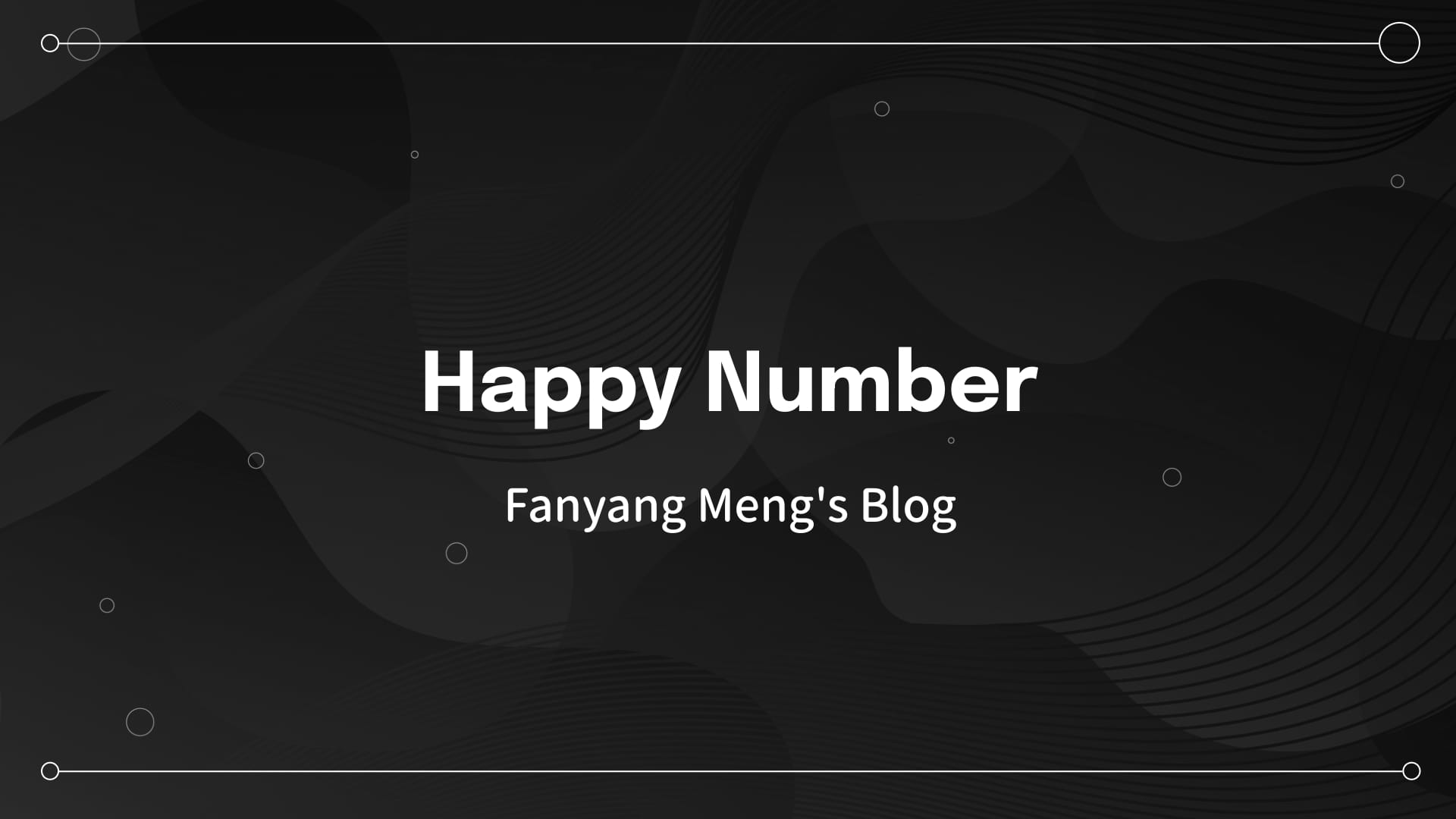
- Problem: Detect cycles in sequences of operations.
- Solution: Use a
set
to track numbers that have already appeared, ensuring efficient cycle detection.
Using Dictionaries as Hash Tables
Dictionaries are highly versatile and ideal when you need to store key-value pairs.
Example 5: Two Sum
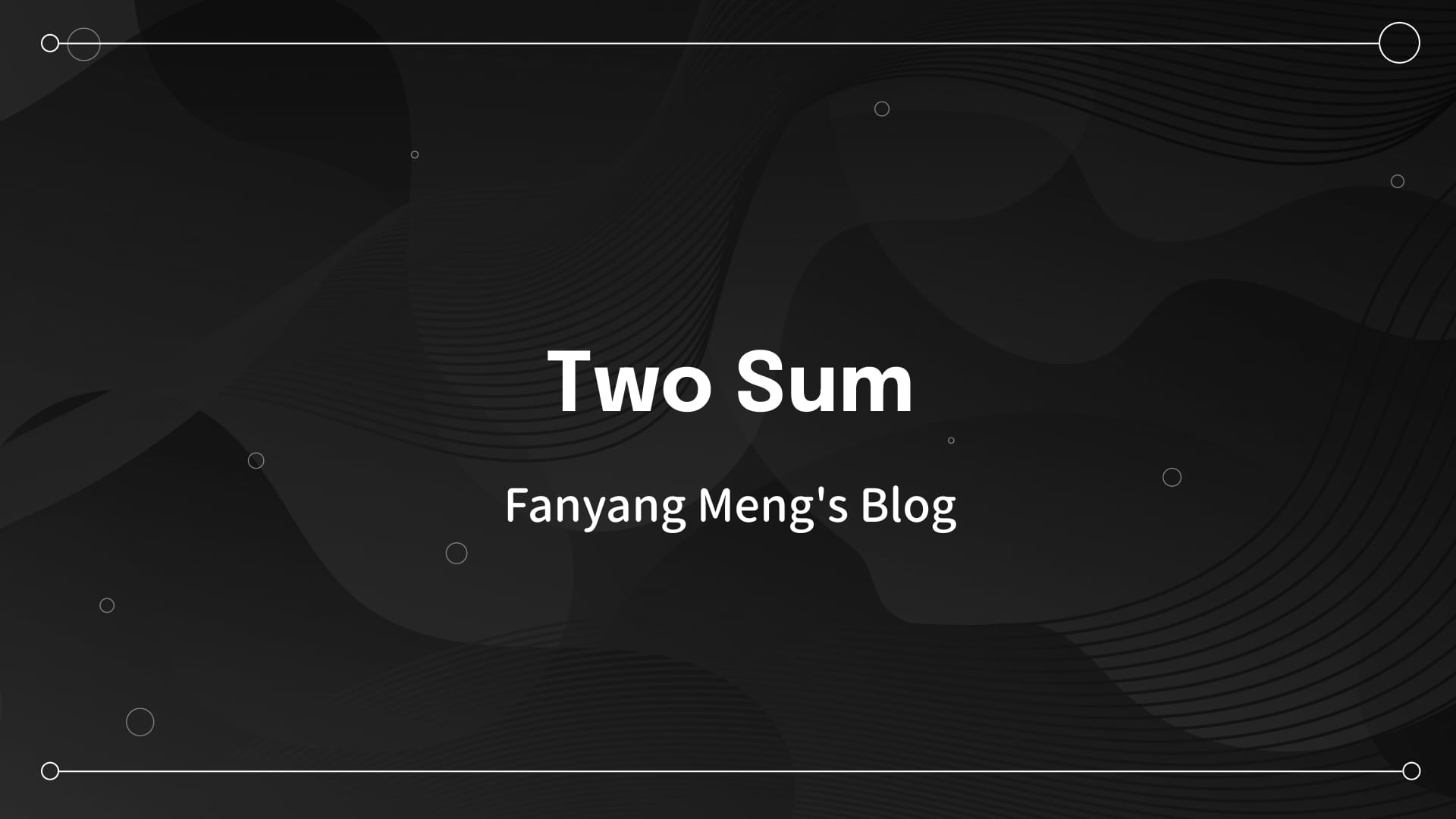
- Problem: Find two numbers in an array that add up to a target.
- Solution: Use a dictionary to store each number’s index, allowing quick lookup for the complement.
Example 6: 4Sum II
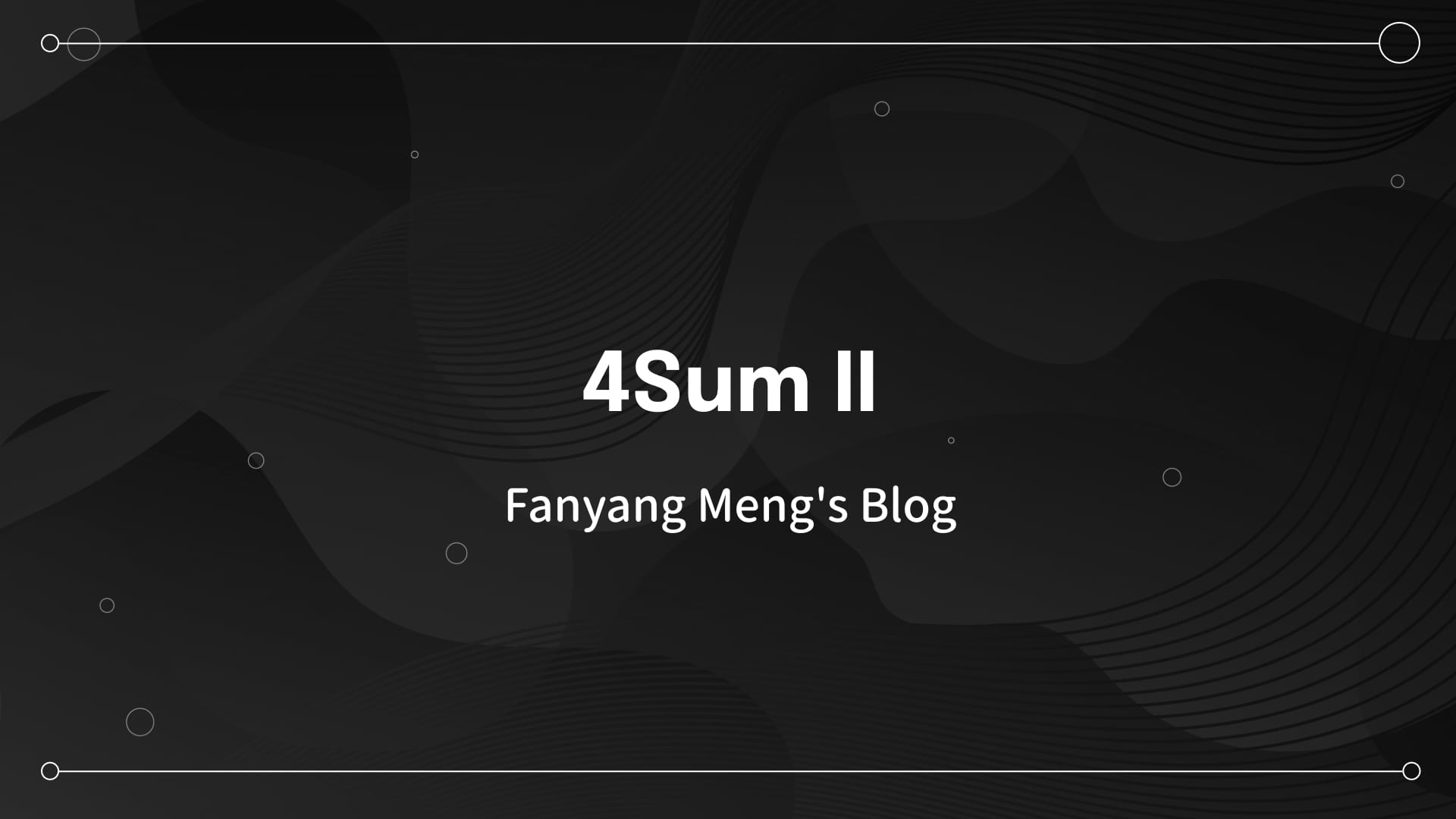
- Problem: Find tuples across four arrays whose elements sum to zero.
- Solution: Use a dictionary to store intermediate sums for efficient lookups.
Comparing with 3Sum/4Sum
Unlike 3Sum or 4Sum, which involve handling duplicates in a single array, 4Sum II uses independent arrays, simplifying the use of dictionaries.
3. Choosing the Right Tool
Here’s a quick comparison of when to use lists, sets, and dictionaries:
Structure | Use When | Examples |
---|---|---|
List | Domain is constrained (e.g., lowercase letters, limited range). | Valid Anagram, Ransom Note |
Set | Domain is large, unknown, or unordered. | Intersection of Two Arrays, Happy Number |
Dictionary | Need to track key-value relationships or handle more complex mappings. | Two Sum, 4Sum II |
4. Conclusion
Hash tables are an indispensable tool for efficient programming. This post outlined their theoretical foundations and demonstrated practical applications using lists, sets, and dictionaries in Python. While dictionaries are highly versatile, understanding when to use lists or sets can significantly optimize your solutions.
Discussion