Fundamentals of Stacks and Queues
Learn the fundamentals of stacks and queues in Python! Discover how to implement stacks using list and deque, and queues with list, collections.deque, queue.Queue, or multiprocessing.Queue. Explore efficient, thread-safe, and scalable solutions for every scenario.
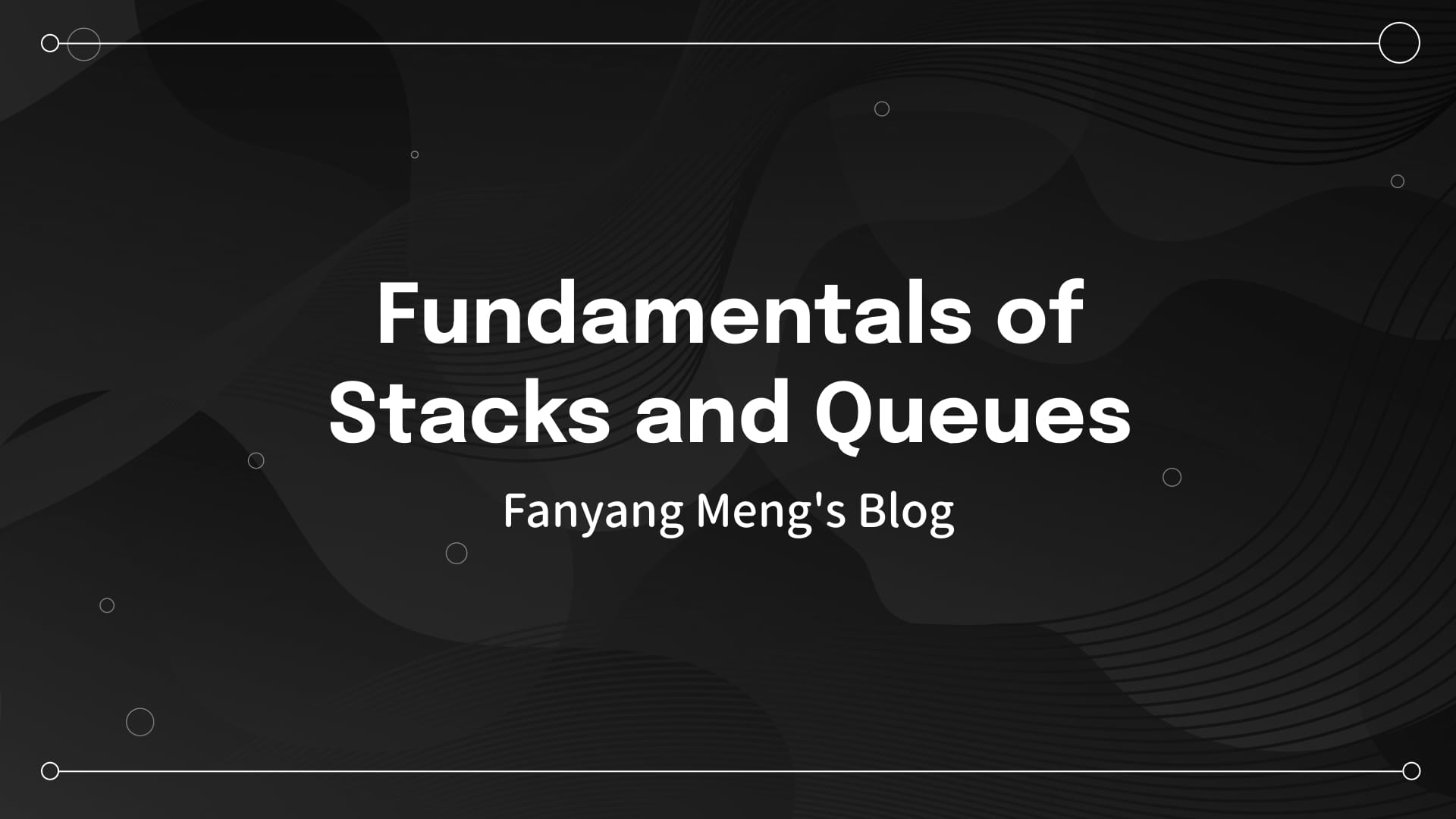
The concepts of stacks and queues should be familiar to most of us. A queue operates on the principle of First In, First Out (FIFO), whereas a stack follows First In, Last Out (FILO).
As illustrated below:
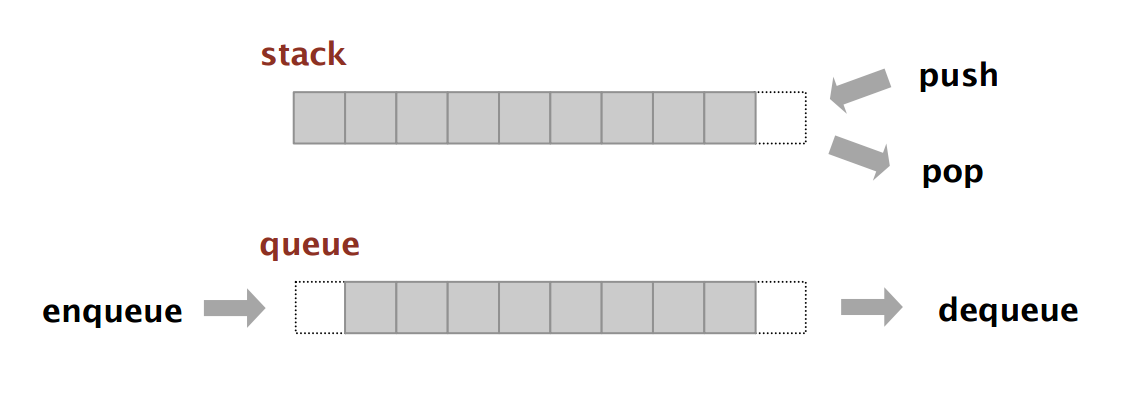
Let me present four key questions about stacks for you to consider. The following explanations will be based on Python, so think about how stacks and queues are implemented in Python:
- Is the
list
in Python inherently a stack structure? - Does Python's built-in
list
or other modules use any specific underlying implementation for stacks? - How are stacks implemented in Python's standard library?
- Can we iterate over a stack in Python?
These are not trivial questions because many people use data structures superficially. However, when we delve into the underlying implementations, things may become less clear. For example, some users might only know that stacks and queues exist as data structures but remain unaware of their underlying mechanics or their relationships with standard libraries like Python's collections
.
In Python, stacks and queues are foundational data structures and can be implemented using the standard list
, or via specialized modules like collections
and queue
. Let’s explore these in detail.
What is a Stack?
A stack operates on the principle of "Last In, First Out" (LIFO), as shown below:
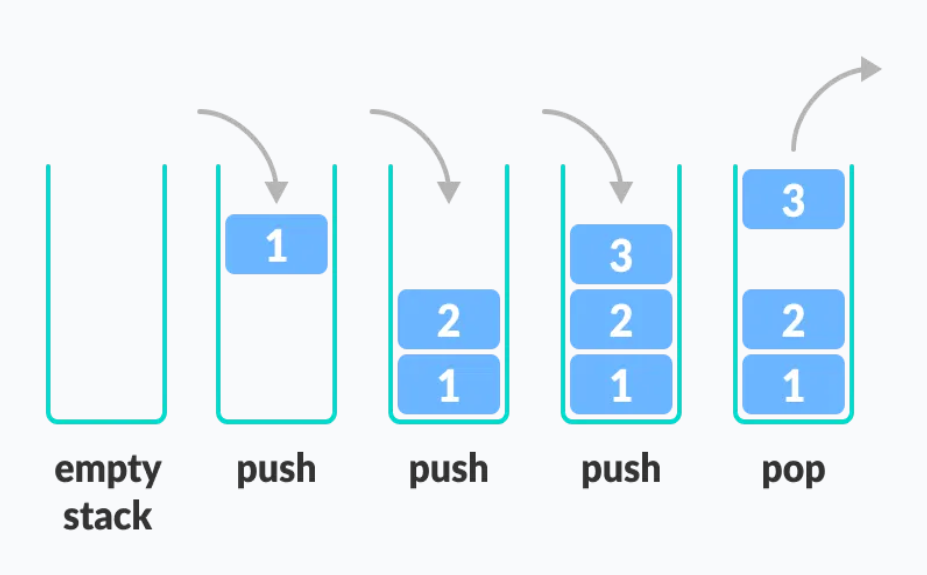
In Python, a stack can be implemented in multiple ways:
1. Using list
Python’s built-in list
data structure supports operations like append()
to push elements and pop()
to remove the last element, making it suitable for stack implementation.
Example:
stack = []
stack.append(1) # Push 1
stack.append(2) # Push 2
stack.pop() # Pop 2 (LIFO)
2. Using collections.deque
While list
can serve as a stack, collections.deque
is preferred for high-performance stack operations because it is optimized for fast append and pop operations from both ends.
Example:
from collections import deque
stack = deque()
stack.append(1) # Push 1
stack.append(2) # Push 2
stack.pop() # Pop 2 (LIFO)
What is a Queue?
A queue operates on the principle of "First In, First Out" (FIFO), as show in below:

In Python, it can also be implemented in various ways.
1. Using list
A queue can be implemented using Python’s built-in list
. You can use the append()
method to enqueue elements and the pop(0)
method to dequeue elements.
Example:
queue = []
# Enqueue
queue.append(1) # Add 1
queue.append(2) # Add 2
# Dequeue
queue.pop(0) # Remove and return 1 (FIFO)
queue.pop(0) # Remove and return 2
Limitations:
Using a list
for a queue is simple but inefficient for large datasets because removing elements from the front (pop(0)
) requires shifting all the subsequent elements, leading to O(n) time complexity for dequeuing.
2. Using collections.deque
Deque is an excellent choice for queues because it supports efficient operations on both ends.
Example:
from collections import deque
queue = deque()
queue.append(1) # Enqueue 1
queue.append(2) # Enqueue 2
queue.popleft() # Dequeue 1 (FIFO)
Advantages:
- Efficient O(1) operations for both enqueue and dequeue.
- A flexible and robust option for implementing queues.
3. Using the queue
module
Python’s queue.Queue
provides thread-safe FIFO queue implementation, suitable for multi-threaded applications.
Example:
from queue import Queue
queue = Queue()
queue.put(1) # Enqueue 1
queue.put(2) # Enqueue 2
# Dequeue
queue.get() # Remove and return 1 (FIFO)
queue.get() # Remove and return 2
Advantages:
- Built-in thread safety.
- Ideal for concurrent programming.
4. Using multiprocessing.Queue
If you are working with multiple processes, you can use the multiprocessing.Queue
, which is specifically designed for inter-process communication.
Example:
from multiprocessing import Queue
queue = Queue()
# Enqueue
queue.put(1) # Add 1
queue.put(2) # Add 2
# Dequeue
queue.get() # Remove and return 1 (FIFO)
queue.get() # Remove and return 2
Advantages:
- Supports process-safe queue operations.
- Useful for multiprocessing applications.
Comparison of Implementations
Implementation | Use Case | Enqueue Complexity | Dequeue Complexity | Thread/Process Safe |
---|---|---|---|---|
list | Simple queues for small data | O(1) | O(n) | No |
collections.deque | General-purpose queues | O(1) | O(1) | No |
queue.Queue | Multi-threaded applications | O(1) | O(1) | Yes |
multiprocessing.Queue | Multi-process applications | O(1) | O(1) | Yes |
Key Points to Remember
- Stacks and queues are essential data structures with well-defined behaviors:
- Stacks follow the LIFO principle (Last In, First Out).
- Queues follow the FIFO principle (First In, First Out).
- Python provides several ways to implement queues, ranging from simple lists to robust and optimized options like
collections.deque
andqueue.Queue
. The best choice depends on your specific requirements:- Use
list
for simplicity in small-scale or learning scenarios. - Use
collections.deque
for efficient general-purpose queues. - Use
queue.Queue
ormultiprocessing.Queue
when thread safety or inter-process communication is required.
- Use
- Python provides flexible and efficient options for these structures, making it easy to use them in various scenarios.
By exploring the underlying principles of these data structures and understanding how they are implemented in Python, you can build a strong foundation in data structures and algorithms. Don't stop at basic usage—go deeper into the mechanics to truly master them.
Discussion