Binary Tree Traversal: A Deep Dive into Recursion
Master binary tree traversal in Python with a systematic approach to recursion. Learn the three essential elements for writing reliable recursive algorithms and implement preorder, inorder, and postorder traversals. Perfect for programmers looking to strengthen their tree manipulation skills.
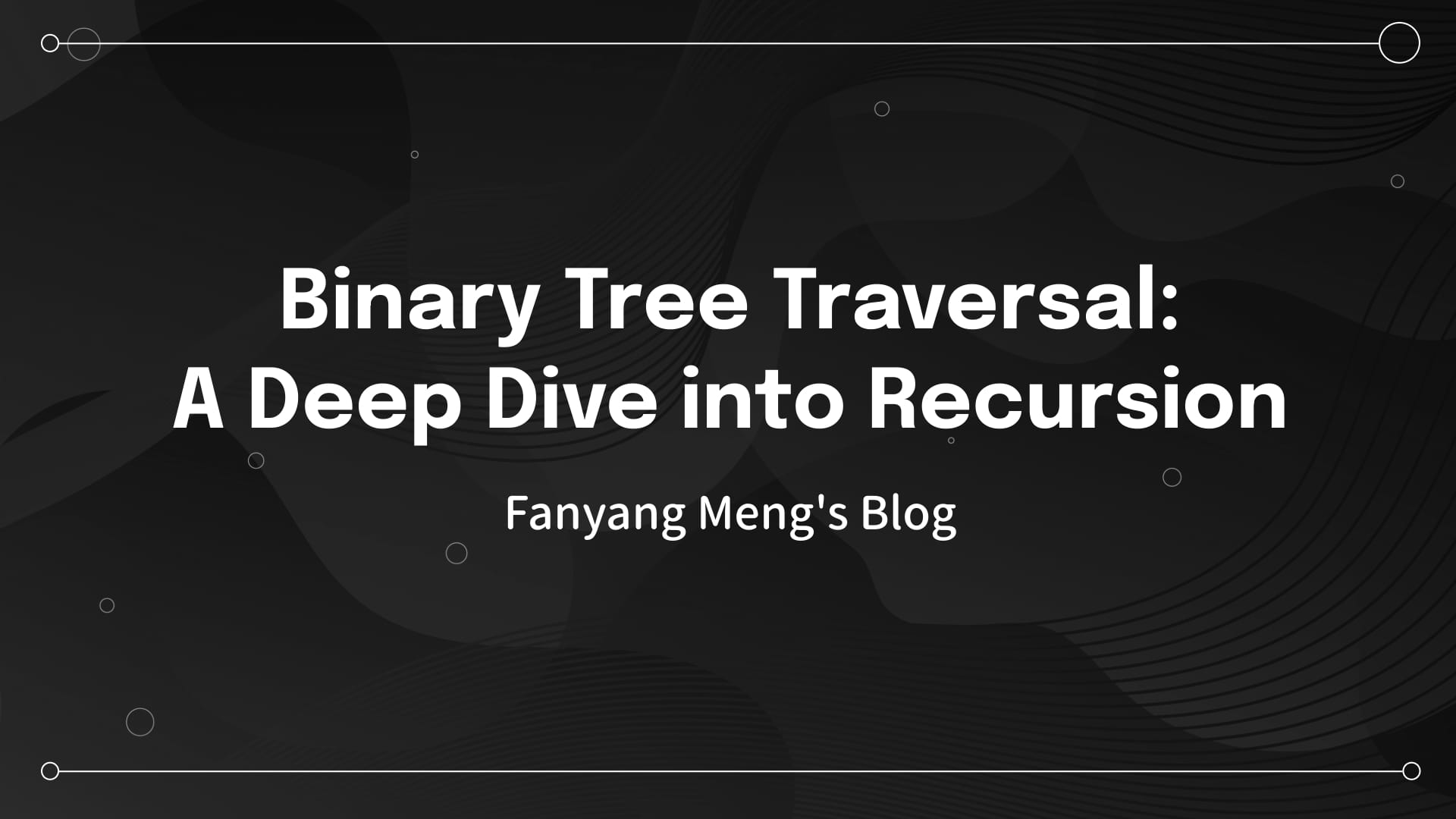
Introduction
Many programmers find themselves in a curious situation with recursive algorithms: while they seem straightforward to understand, implementing them correctly can be surprisingly challenging. This is particularly true when working with binary trees, where recursive traversal is a fundamental operation.
This blog post aims to transform your understanding of recursive tree traversal from intuitive guesswork into a systematic approach. We'll focus on developing a reliable methodology that you can apply to any recursive tree problem.
The Three Essential Elements of Recursion
Before diving into specific implementations, let's establish three crucial elements that every recursive algorithm must have. By following these elements consistently, you can write correct recursive solutions with confidence:
- Define Function Parameters and Return Values: Carefully identify which parameters your recursive function needs to process and what it should return. This forms the foundation of your recursive implementation.
- Establish Termination Conditions: Without proper termination conditions, recursive functions can cause stack overflow errors. The program uses a stack to store information about each recursive call, and without proper termination, this stack will eventually overflow.
- Implement Single-Level Recursive Logic: Define what needs to happen at each recursive step. This is where you'll implement the core logic and make recursive calls.
Let's apply these principles to binary tree traversal algorithms.
Implementing Preorder Traversal
If you do not know what is preorder traversal, you can find it in my previous blog post:
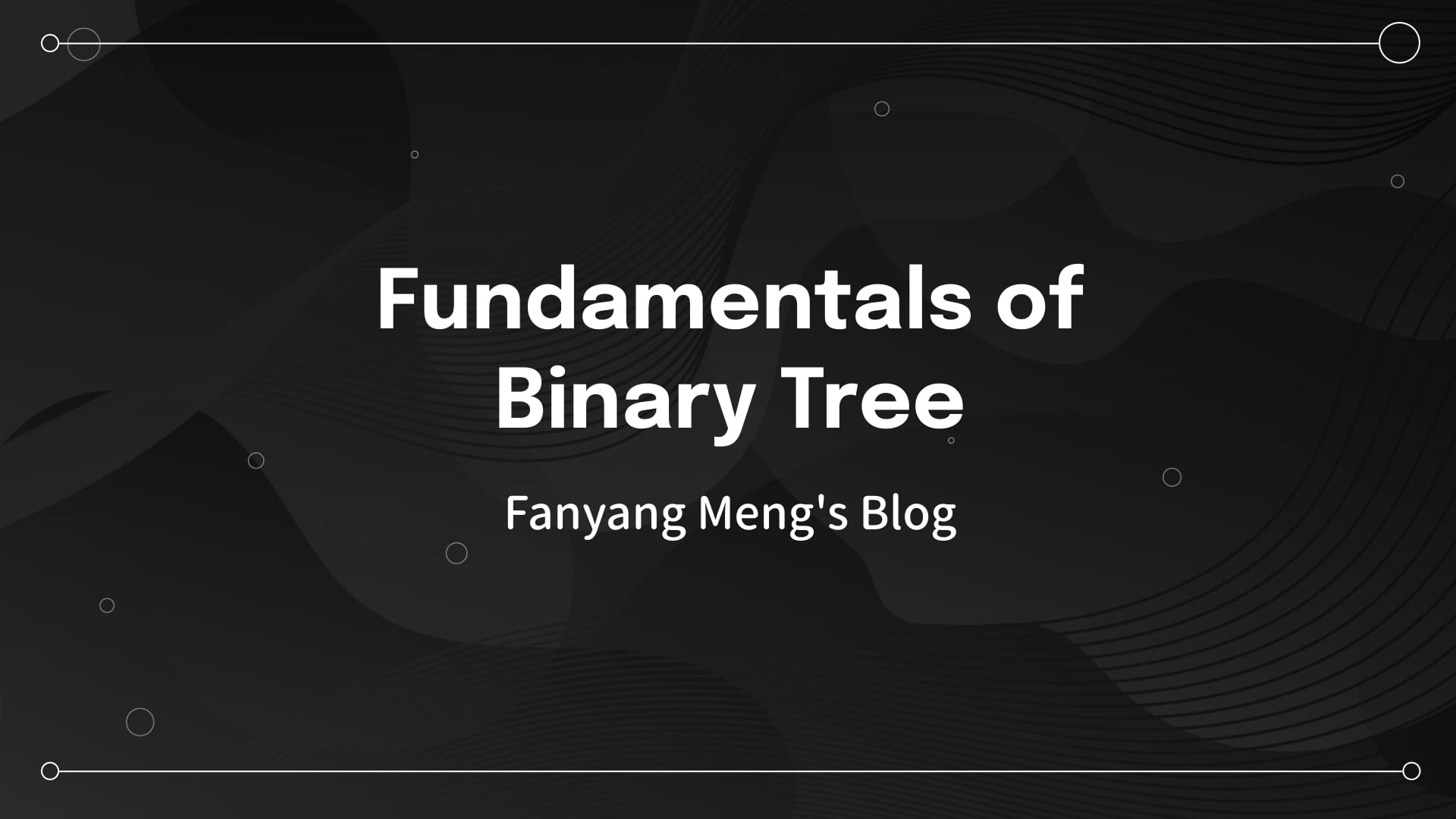
Let's start with preorder traversal as an example:
- Defining Function Parameters and Return Values:
def preorder_traversal(root: TreeNode) -> List[int]:
result = []
def dfs(node: TreeNode) -> None:
# Using inner function for cleaner implementation
if node is None:
return
dfs(root)
return result
- Establishing Termination Conditions:
if node is None:
return
- Implementing Single-Level Logic:
result.append(node.val) # Process current node
dfs(node.left) # Process left subtree
dfs(node.right) # Process right subtree
Here's the complete implementation:
class Solution:
def preorder_traversal(self, root: TreeNode) -> List[int]:
result = []
def dfs(node: TreeNode) -> None:
# Termination condition
if node is None:
return
# Process current node (preorder: root -> left -> right)
result.append(node.val)
dfs(node.left)
dfs(node.right)
dfs(root)
return result
Inorder and Postorder Traversal
Once you understand preorder traversal, implementing inorder and postorder traversal becomes straightforward. The only difference is the order of operations:
class Solution:
def inorder_traversal(self, root: TreeNode) -> List[int]:
result = []
def dfs(node: TreeNode) -> None:
if node is None:
return
# Inorder: left -> root -> right
dfs(node.left)
result.append(node.val)
dfs(node.right)
dfs(root)
return result
def postorder_traversal(self, root: TreeNode) -> List[int]:
result = []
def dfs(node: TreeNode) -> None:
if node is None:
return
# Postorder: left -> right -> root
dfs(node.left)
dfs(node.right)
result.append(node.val)
dfs(root)
return result
Practice Problems
To reinforce your understanding, try solving these LeetCode problems:
- Binary Tree Preorder Traversal (144)
- Binary Tree Inorder Traversal (94)
- Binary Tree Postorder Traversal (145)
Next Steps
While recursive solutions are elegant and intuitive, they're not the only way to traverse binary trees. In real-world applications, you might need to implement iterative solutions using stacks or queues, especially when dealing with very deep trees where recursive solutions might cause stack overflow.
Understanding these recursive patterns forms the foundation for more complex tree operations. Once you master these basics, you'll be better prepared to tackle more challenging tree-related problems.
Remember: the key to writing correct recursive solutions is not relying on intuition alone, but following a systematic approach using the three essential elements we discussed.
Discussion